Table of Contents
In Python, floor division is a powerful operator that allows you to divide two numbers and return the largest integer less than or equal to the result. Unlike regular division, which can produce a floating-point number, floor division simplifies the output by discarding the decimal portion. This makes it particularly useful in scenarios where you need whole numbers, like indexing, counting, or working with discrete data.
In this guide, we will explore how floor division works in Python, demonstrate its syntax, and provide practical examples to illustrate its use in various applications.
How does Floor Division Work?
Floor division is a division operation that returns the largest integer that is less than or equal to the result of the division. In Python, it is represented by the double forward slash //
. This operator ensures that any fractional part of the result is discarded, yielding a whole number.
For example, when you divide 7 by 2 using floor division, the result is 3, as it rounds down to the nearest integer. This makes floor division particularly useful in scenarios where you need integer results.
Code Implementation
In Python, there are primarily two methods to perform floor division: using the floor division operator (//
) and using the math.floor()
function along with regular division. Let’s explore these two methods in more detail.
1. Floor Division Operator (//
)
The most straightforward way to perform floor division is by using the //
operator. This operator divides two numbers and automatically rounds down to the nearest whole number. Let’s see some code examples:
result = 7 // 2
# 7 / 2 = 3.5
# .5 is truncated
# Output: 3
result = -10 // 3
# -10 / 3 = -3.333...
# .333... is truncated
# Output: -4
result = 13 // -2
# 13 / -2 = -6.5
# .5 is truncated
# Output: -7
result = -9 // -4
# -9 / -4 = 2.25
# .25 is truncated
# Output: 2
2. math.floor()
Function
You can also achieve floor division by first performing regular division and then applying the math.floor()
function to round down to the nearest integer. Let’s see some code examples:
import math
division = 7 / 2
result = math.floor(division)
print(result) # Output: 3
result = math.floor(10 / 3)
print(result) # Output: 3
Conclusion
Floor division is an operation in Python that returns the largest integer less than or equal to the result of dividing two numbers. It is particularly useful for obtaining whole numbers when performing calculations. To implement floor division in Python, we can use these two methods:
- Floor Division Operator (
//
):
Syntax:result = a // b
math.floor()
Function:
Syntax:result = math.floor(a / b)
In the context of blockchain development, Python’s versatility and robust libraries make it an excellent choice for building and interacting with blockchain systems. For instance, floor division can be useful in blockchain applications when calculating block sizes, transaction fees, or distributing resources among nodes, ensuring that results are always whole numbers.
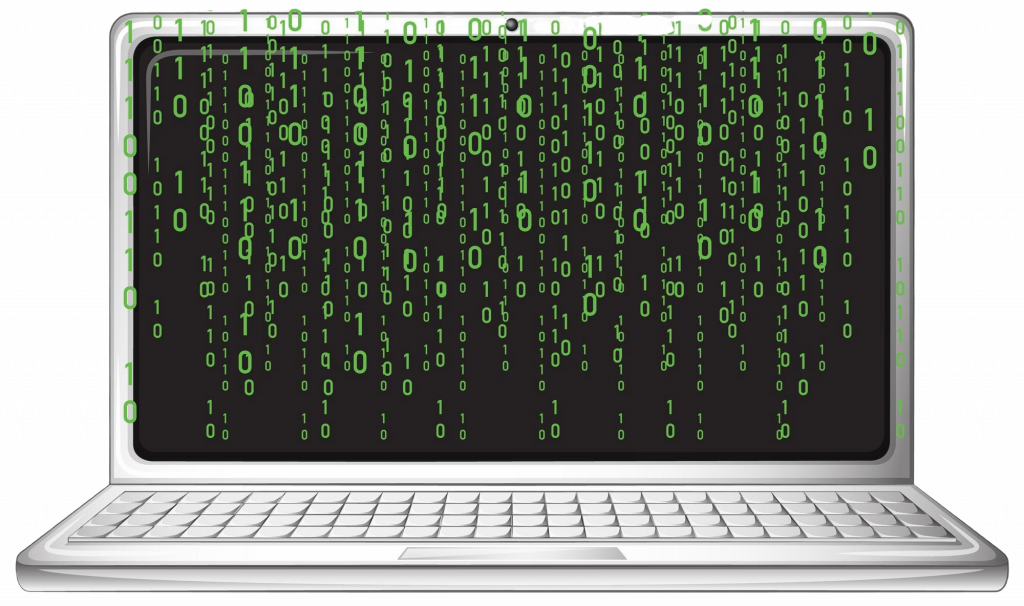
To learn more about why Python is a powerful tool for blockchain projects, check out our article, 7 Reasons Why You Should Develop a Blockchain Using Python, which explores the many advantages of using Python in this field.
FAQs
What is the difference between floor division (//
) and regular division (/
) in Python?
The key difference between floor division (//
) and regular division (/
) in Python is how they handle the result. Floor division returns the largest integer less than or equal to the division result, effectively truncating any decimal portion.
For example, 7 // 2
results in 3
, whereas 7 / 2
results in 3.5
. Use floor division when you need an integer result.
When should I use math.floor()
instead of math.round()
?
Use math.floor()
when you want to round down to the nearest integer, regardless of the decimal value. For example, math.floor(3.7)
returns 3
. In contrast, math.round()
rounds to the nearest integer, meaning math.round(3.7)
returns 4
, and math.round(3.4)
returns 3
.
Choose math.floor()
when you need consistent downward rounding and math.round()
when you want standard rounding behavior.
How can floor division be useful in blockchain development?
Floor division can be particularly useful in blockchain development for calculating whole numbers required in various scenarios. For instance, it can help determine how many transactions can fit in a block, calculate fees that must be rounded down to avoid fractional values, or evenly distribute resources among nodes in a network. Using floor division ensures that all calculations yield integer results, which are often essential in these contexts.