Table of Contents
Evolution of Programming Languages
The evolution of programming paradigms can be traced through several key stages.
Procedural Programming
Procedural programming emerged in the 1950s and 1960s and is one of the earliest programming paradigms. It organizes code into procedures or functions that operate on data. Programs are structured as a sequence of steps or instructions, focusing on how tasks are performed.
Limitations: While procedural programming works well for smaller programs, it becomes challenging to manage and maintain as programs grow larger and more complex. Code duplication, tightly coupled functions, and difficulties in understanding large codebases are common issues.
Modular Programming
In response to the limitations of procedural programming, modular programming was introduced. It encourages dividing programs into smaller, independent modules that perform specific tasks. Each module can be developed and tested independently, improving code organization and reducing complexity.
Limitations: While modular programming is useful for organizing code it has some drawbacks. It doesn’t provide a natural way to protect and manage data, making it easy for different parts of a program to unintentionally interfere with each other. It also has difficulty representing complex real-world concepts, and modules can become too dependent on each other, making the code harder to update and maintain. These challenges made it clear that a more advanced approach was needed for larger software projects – enter Object Oriented Programming (OOP).
Object Oriented Programming
Structure
The key components of object-oriented programming are:
- Classes are user-defined data types that serve as blueprints for creating objects. They include attributes (properties) and methods (functions) that define the behaviors of the objects.
Class: Car
Attributes: make, model, year, color
Methods: start_engine(), accelerate(), brake()
- Objects are instances of a class, representing real-world entities or abstract concepts. They are created based on the class definition.
Object: my_car
Attributes: make = "Toyota", model = "Corolla", year = 2021, color = "blue"
Methods: my_car.start_engine(), my_car.accelerate(), my_car.brake()
- Methods are functions defined within a class that describe the behaviors of objects. They start with a reference to an instance object and enable functionality to be reused or encapsulated.
- Attributes are characteristics or properties of an object that define its state. Class attributes belong to the class itself, while instance attributes belong to specific objects.
Principles of Object Oriented Programming
Abstraction
Abstraction allows us to design classes with clean, high-level interfaces that are easy to use while hiding the complexity of the implementation behind the scenes. This simplifies interaction with complex systems by exposing only the essential features, making it easier to understand and use classes without worrying about the underlying details of how they work.
Encapsulation
Encapsulation bundles data (attributes) and behavior (methods) into a single class, keeping important information private while exposing only specific functions or methods. This approach protects data integrity by restricting direct access and modifications, enhancing security, and avoiding unintended data corruption.
Inheritance
Inheritance allows a new class (subclass) to inherit properties and methods from an existing class (superclass), enabling code reuse and the creation of a class hierarchy. This mechanism facilitates the reuse of common logic, reduces development time, and ensures accuracy by promoting a structured hierarchy and more thorough data analysis.
Polymorphism
Polymorphism allows methods to perform different tasks based on the object they act upon, even if they share the same name. This feature enables the use of a common interface for various implementations, allowing objects to share behaviors and take on multiple forms. By utilizing method overriding in subclasses, polymorphism reduces code duplication and ensures that different types of objects can interact through the same interface, adapting their functionality as needed.
Let’s try to understand the important features of object oriented programming using a coded example in Python.
# Base class (Superclass)
class Book:
# Constructor (Initialization)
def __init__(self, title):
self.__title = title # Private attribute (Encapsulation)
# Method (Encapsulation)
def borrow(self):
print(f"{self.__title} has been borrowed.")
# Subclass that extends Book (Inheritance)
class EBook(Book):
def __init__(self, title, file_size):
super().__init__(title) # Call the superclass constructor
self.__file_size = file_size # New private property specific to EBook
# Overriding the borrow method (Polymorphism)
def borrow(self):
print(f"{self._Book__title} (E-Book, {self.__file_size}MB) has been borrowed.")
# Main function to demonstrate OOP concepts
if __name__ == "__main__":
# Create an instance of Book (Abstraction)
physical_book = Book("The Great Gatsby")
# Create an instance of EBook (Inheritance)
ebook = EBook("1984", 1.5)
# Demonstrate polymorphism
physical_book.borrow() # Calls the borrow method from Book
ebook.borrow() # Calls the overridden borrow method from EBook
Expected Output

Let’s look at a thorough explanation of the code which demonstrates the principles of OOP.
Abstraction
The Book
and EBook
classes are abstractions that represent different types of books. By creating the Book
and EBook
classes, the code abstracts the concept of a book into objects that have specific properties and methods. Users of these classes don’t need to know how these properties and methods are implemented; they only need to know how to interact with the objects.
Encapsulation
In the Book
class, the __title
attribute is marked as private by using double underscores. The double underscore prefix triggers name mangling, which changes the name of the attribute internally to _ClassName__AttributeName
. This makes it more difficult (but not impossible) to access these attributes directly from outside the class. The attribute __title
in the Book
class is accessed within the class through methods like borrow()
. In the EBook
class, the overridden borrow()
method accesses the mangled name _Book__title
to correctly reference the private attribute from the superclass.
Inheritence
The EBook
class inherits from the Book
class. The EBook
class is a specialized version of the Book
class. It inherits the title
attribute and the borrow()
method from Book
, allowing it to reuse and extend the functionality of Book
. The EBook
class also introduces a new attribute, file_size
, specific to e-books.
Polymorphism
The borrow()
method is overridden in the EBook
class.The EBook
class provides its own implementation of the borrow()
method, which includes additional information about the file size. When borrow()
is called on an EBook
instance, the overridden method is executed, demonstrating polymorphism. This allows the same method name (borrow
) to exhibit different behaviors depending on the object (Book
or EBook
).
Summary:
- Encapsulation: The
__title
and__file_size
attributes are made private, protecting them from unintended external access and manipulation. - Inheritance: The
EBook
class inherits and reuses functionality from theBook
class. - Polymorphism: The
borrow()
method is overridden in theEBook
class, demonstrating how the same method can behave differently based on the object. - Abstraction: The
Book
andEBook
classes abstract the concept of a book, allowing interaction with complex data structures in a simplified manner.
Implementational Benefits
1. Modularity
Object oriented programming promotes modularity by organizing code into distinct classes, each representing a specific entity or concept. These classes encapsulate both data and behavior, allowing developers to create these units that interact with each other through well-defined interfaces. This approach makes it easier to understand, develop, and test individual components of a system independently since developers can work on different parts of a project simultaneously without causing conflicts, which enhances collaboration and productivity.
Example: In a library management system, you might have separate classes for Book
, Member
, and Loan
. The Book
class handles book details like title and author, the Member
class manages user information, and the Loan
class oversees the borrowing and returning process. Each class operates independently but interacts with others through defined methods, making the system easier to manage and extend .
2. Code Reusability
Object oriented programming enables code to reuse through two fundamental concepts: inheritance and polymorphism. Inheritance allows the subclass to inherit properties and methods from the superclass, reducing the need to write duplicate code. Polymorphism enables a single interface to represent different underlying forms (data types), allowing the same code to work with different objects.
Example: Imagine you have a superclass Shape
with methods like draw()
and resize()
. Subclasses such as Circle
, Rectangle
, and Triangle
can inherit these methods and implement their specific versions. Polymorphism allows you to call the draw()
method on any object of type Shape
, whether it’s a Circle
, Rectangle
, or Triangle
, enabling the same piece of code to handle different shapes effectively .
3. Ease of Maintenance
Object oriented programming makes maintenance easier by organizing code into classes that encapsulate specific functionalities. This separation of concerns allows developers to update or modify one part of the code without affecting others. Additionally, because classes are designed to be reusable, any bug fixes or updates in a superclass automatically propagate to all its subclasses, reducing the overall effort.
Example: Consider an e-commerce application with a PaymentProcessor
class that handles payment methods. If a new payment gateway needs to be integrated, you can modify or extend the PaymentProcessor
class without changing the rest of the application’s code. This modular approach ensures that updates are localized and don’t impact unrelated parts of the system.
4. Improved Productivity
Object oriented programming enhances developer productivity by providing a clear and organized way to structure code. It also encourages the use of design patterns, which are proven solutions to common problems, further speeding up the development process. As a result, developers can focus more on solving the business problem rather than worrying about the underlying code structure.
Example: In a project management tool, teams can work on different modules such as task management, user management, and reporting. Each team develops its module independently, using existing classes and components. This parallel development speeds up the project timeline, and the modular structure allows for easier integration and testing of new features .
Common OOP Languages
Object oriented programming is implemented in various programming languages, each with its unique approach to applying OOP principles.
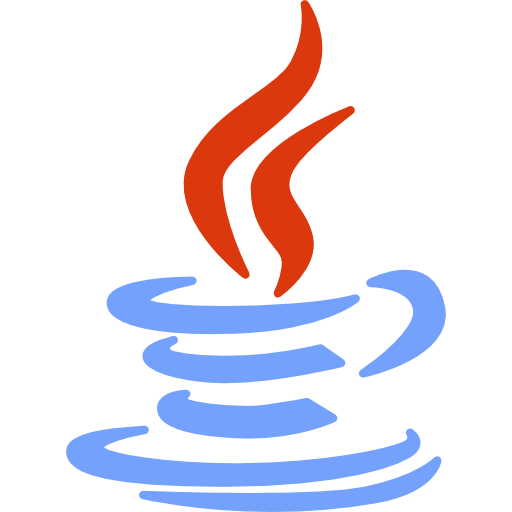
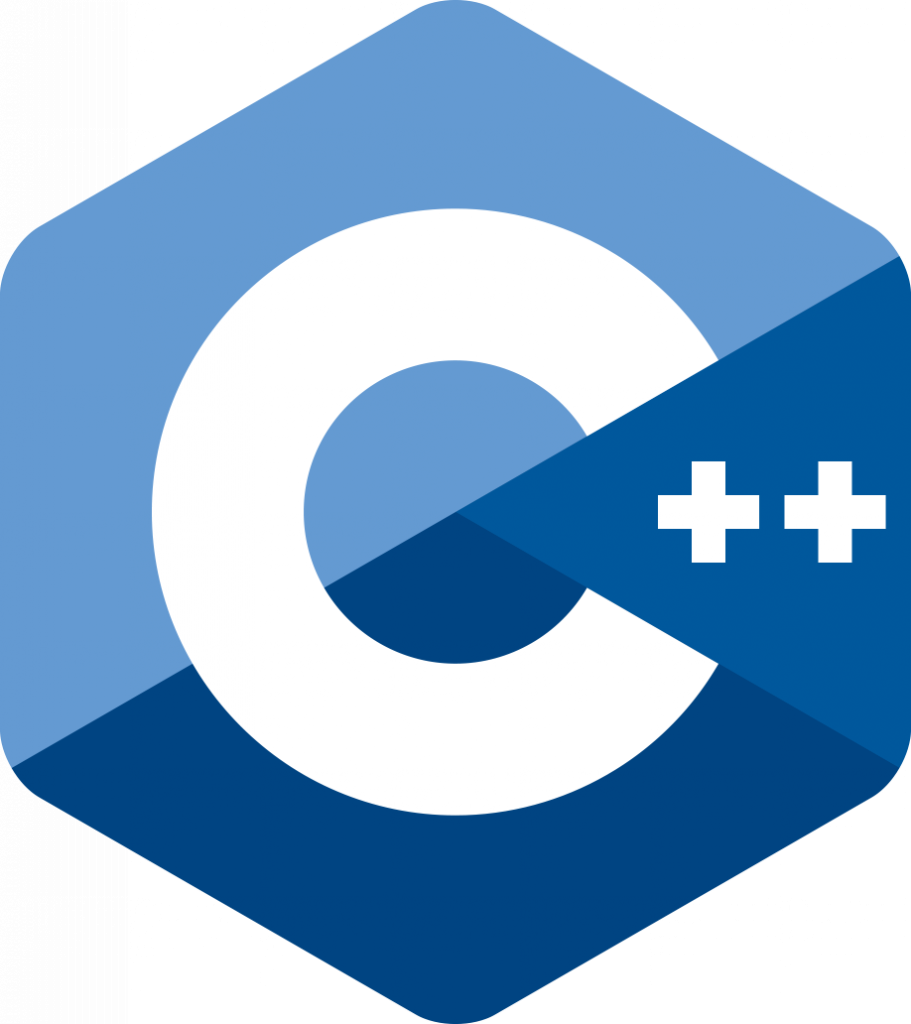
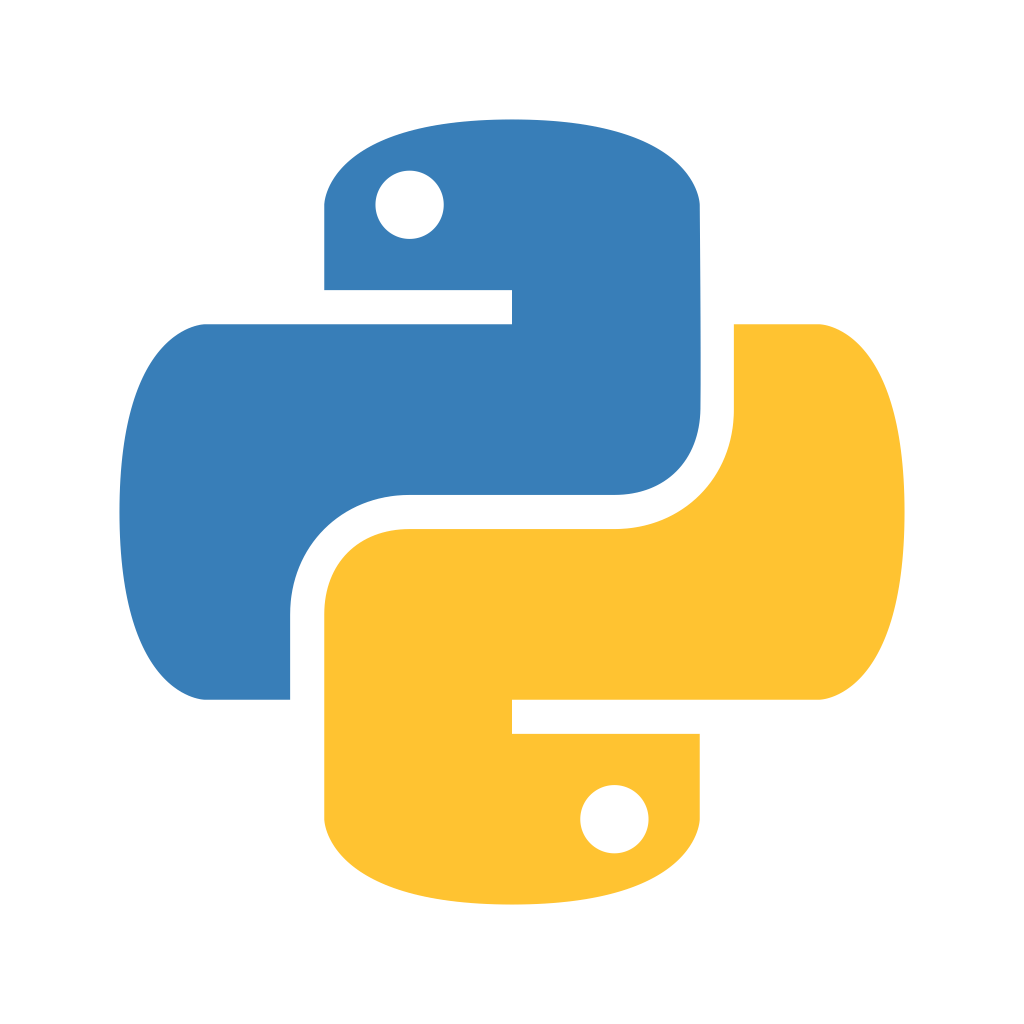
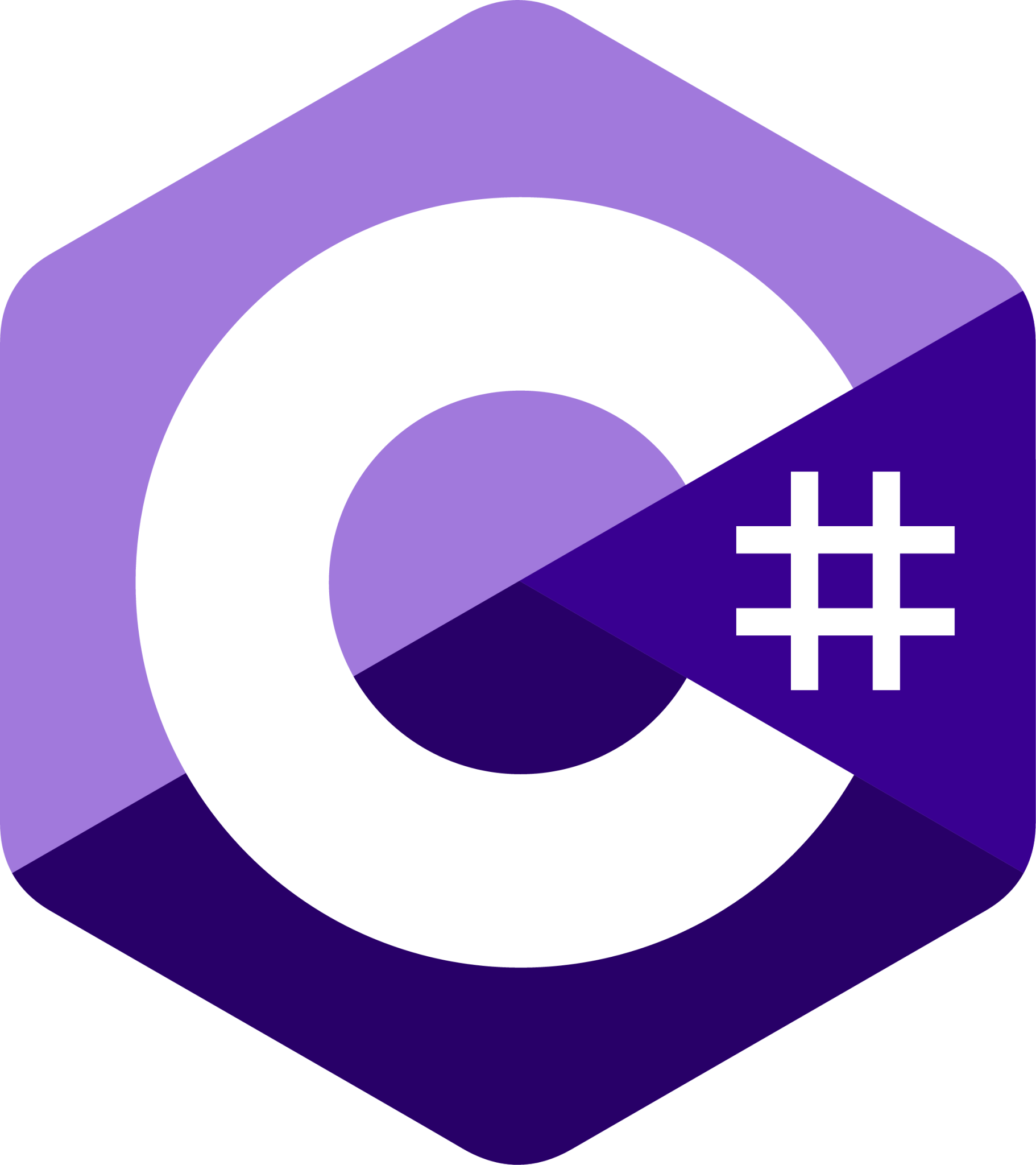
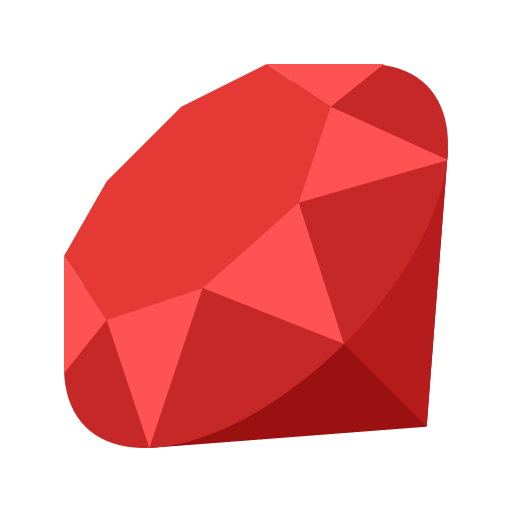
- Java:
Java is a fully object-oriented language where everything is treated as an object, except for primitive data types. It’s known for its platform independence, encapsulation through classes, and robust inheritance mechanisms. Java’s emphasis on object-oriented principles makes it a staple in enterprise-level applications, Android development, and large-scale systems. - C++:
C++ extends the procedural language C with object-oriented features. It offers fine-grained control over system resources while supporting OOP concepts like classes and inheritance. C++ is widely used in performance-critical applications, such as game development, operating systems, and real-time simulation, due to its ability to blend low-level programming with OOP. - Python:
Python is a versatile language that embraces OOP principles while being easy to learn and use. It allows for a more flexible and less rigid application of OOP, making it popular in fields like web development, data science, and machine learning. Python’s dynamic typing and straightforward syntax make it a go-to language for both beginners and experienced developers. - C#:
Developed by Microsoft, C# is deeply integrated with the .NET framework and follows OOP principles closely. It is commonly used for developing Windows applications, web services, and games (especially with Unity). C# combines the power of C++ with the ease of Java, making it a strong choice for general-purpose programming. - Ruby:
Ruby is known for its elegant syntax and focus on simplicity, making it highly suitable for web development. It is fully object-oriented, meaning that even primitive data types are objects. Ruby’s OOP implementation encourages clean and maintainable code, which is why it’s favored in frameworks like Ruby on Rails for developing web applications.
These languages, while different in syntax and use cases, all utilize the core principles of OOP to help developers create modular, reusable, and scalable code.
OOP’s Role in Blockchain Technology
In blockchain development, object oriented programming allows developers to create objects that represent key components of a blockchain network, such as transactions, blocks, nodes, and smart contracts. This modularity not only simplifies the process of building and maintaining blockchain systems but also makes it easier to introduce new features and functionalities without disrupting the existing architecture.
Moreover, OOP supports the implementation of design patterns like the observer pattern, which is particularly valuable in creating event-driven systems within blockchain applications. This enables blockchain networks to react to changes in real time, ensuring that applications remain responsive and scalable as they grow.
By leveraging OOP, developers can build more robust and adaptable blockchain solutions, making it easier to integrate blockchain technology into existing systems and respond to the evolving demands of decentralized applications. The use of OOP thus contributes significantly to the flexibility and resilience of blockchain technology.