Table of Contents
Web3 libraries serve as an essential tool in the field of web3 development, enabling developers to not just create but also manage decentralized applications (dApps) that operate on blockchain technology. These libraries provide a variety of tools and frameworks that simplify the interaction between the users of these applications and the underlying blockchain network. They act as a middle layer between the user and the network in general. These web3 libraries ease the implementation of the dApp by the developers by offering developers a more accessible and manageable means to build, deploy, and interact with smart contracts and other features on the network.
Web3.js
web3.js is the most widely used JavaScript library for interacting with Ethereum and EVM-compatible blockchains. It provides a large variety of tools and features for building decentralized applications.
Key Features:
- It supports a wide range of Ethereum and EVM-related functionalities which include smart contract interactions, transactions, and accessing data onchain.
- It provides a consistent and intuitive API, making it easier for developers to work with Ethereum and EVM-based blockchains.
- It is known for its modular design, allowing developers the ease to customize and extend the library as per the need of the application.
Implementation of web3.js library
import Web3 from 'web3';
// Initialize Web3
const web3 = new Web3('https://mainnet.infura.io/v3/your-api-key');
// Create contract instance
const contract = new web3.eth.Contract(ABI, contractAddress);
// Call contract method
const result = await contract.methods.getValue().call();
console.log('Contract value:', result);
// Send transaction
const tx = await contract.methods.setValue(newValue).send({
from: userAddress,
gas: 2000000,
});
Ethers.js
Another very popular name among web3 libraries is the ether.js library for Ethereum and EVM-related interactions. It is known for its comprehensive functionality, ease of implementation, and a strong focus on security.
Key Features:
- It provides a complete set of tools for Ethereum development which includes features like transaction handling, interacting with smart contracts, and wallet management.
- It emphasizes highly on security and stability, with a focus on minimizing vulnerability attacks like 51%, re-entrancy attacks, and so on.
- It also offers modular design like web3.js, allowing developers to include only the necessary components in their applications as per the requirement.
Implementation of ethers.js library
// Initialize provider
const provider = new ethers.providers.JsonRpcProvider('https://mainnet.infura.io/v3/your-api-key');
// Connect to contract
const contract = new ethers.Contract(address, abi, provider);
// Read contract data
const data = await contract.getData();
// Connect with signer for transactions
const signer = provider.getSigner();
const contractWithSigner = contract.connect(signer);
await contractWithSigner.setData(newValue);
Ankr
Ankr is an all-in-one web3 development tool, it isn’t just a library but also offers a wide set of tools and features to build and manage applications across multiple blockchain ecosystems and not just on Ethereum. It provides a versatile platform for deploying applications focussing on scalability, security, and performance of the dApp.
Key Features:
- It provides multi-chain support for popular networks like Ethereum, Polygon, and more.
- It takes care of infrastructure-related services that include load-balanced RPC nodes and hosting solutions.
- It is compatible with multiple blockchain networks, thus it provides cross-chain communication and message-passing protocols in an application.
Implementation of Ankr library
// Initialize Ankr provider
const provider = new AnkrProvider('your_api_key');
// Example of connecting to multiple chains
const ethNode = provider.connect('eth');
const polygonNode = provider.connect('polygon');
// Making a simple RPC call
const balance = await ethNode.getBalance('your_wallet_address');
console.log('ETH Balance:', ethers.utils.formatEther(balance));
libp2p
libp2p library is a modular networking stack perfect for building peer-to-peer applications and is widely used by various protocols like IPFS, Polkadot, and more. It provides a wide variety of tools for developers to be able to create robust and adaptable peer-to-peer networks.
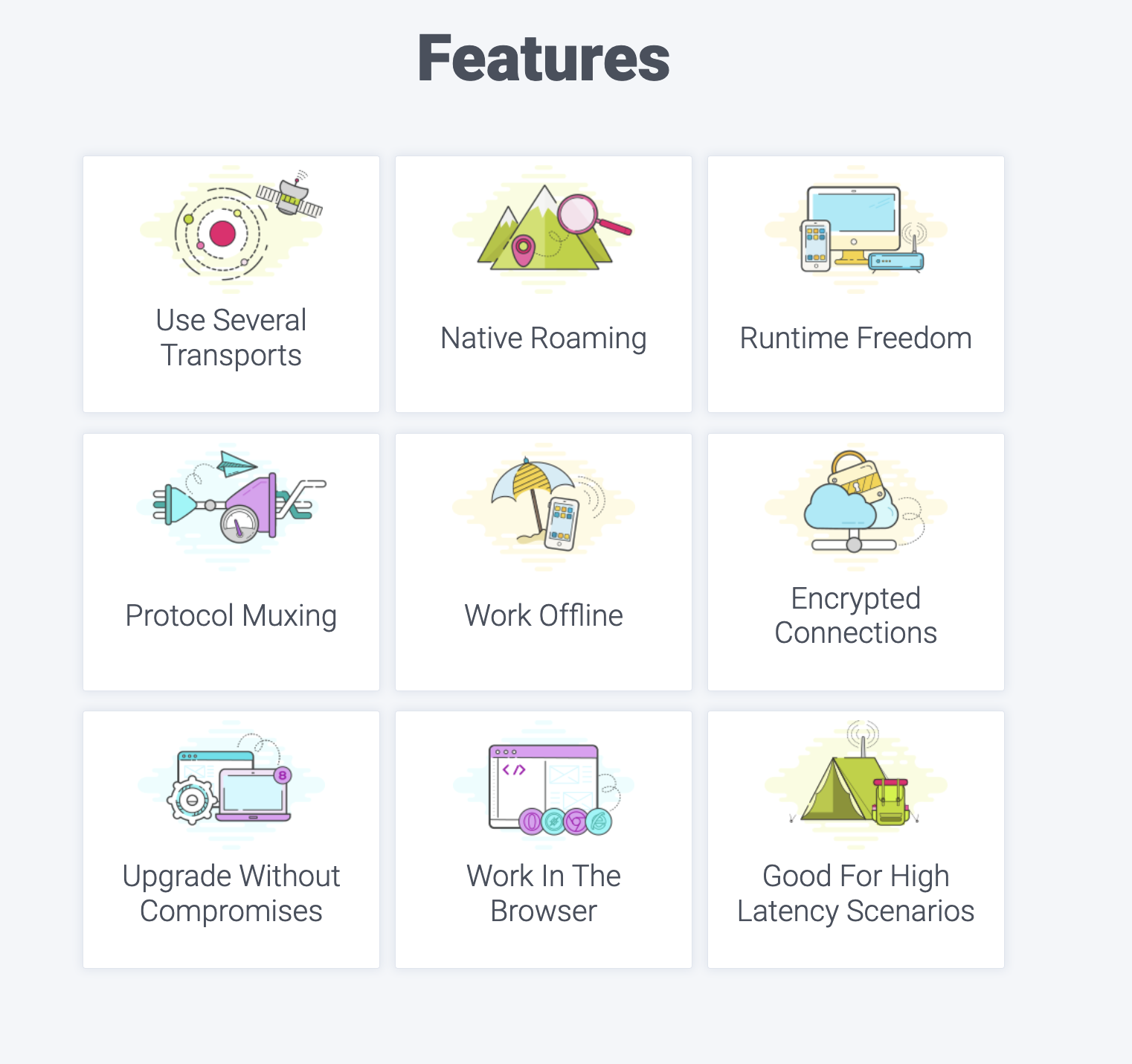
Key Features:
- It provides support for various programming languages including JavaScript, Rust, and Go.
- It has a modular architecture providing encrypted connections, and feasibility for high latency scenarios.
- It has efficient peer discovery and routing mechanisms, also providing runtime freedom and protocol muxing.
// Basic libp2p node setup
const node = await Libp2p.create({
addresses: {
listen: ['/ip4/0.0.0.0/tcp/0']
},
modules: {
transport: [TCP],
connEncryption: [NOISE],
streamMuxer: [MPLEX]
}
});
// Start the node
await node.start();
console.log('Node started with ID:', node.peerId.toString());
Light.js
It is a lightweight reactive JavaScript library for Ethereum and EVM-compatible chains. It is widely used for building clients and mobile applications.
Key Features:
- Supports filtering and caching of data to further improve the performance of the application.
- It is designed with a focus on performance optimization, reducing the need for synchronization of a full node on Ethereum.
- It provides a high-level API for common Ethereum operations, such as querying balances and subscribing to events.
// Initialize light.js
const light = new Light();
// Subscribe to latest block
light.blocks$.subscribe(block => {
console.log('New block:', block.number);
});
// Watch account balance
light.balanceOf$('0x...').subscribe(balance => {
console.log('Balance changed:', balance);
});
Web3j
It is based on the programming language Java for Ethereum interaction, very widely used among Android developers. It is especially popular among Java and Android developers due to its ease of implementation and effectiveness with the application.
Key Features:
- It offers a reactive programming model using RxJava for efficient data handling in the applications.
- It provides a large set of tools for working with smart contracts, handling transactions, and accessing data on-chain.
- It supports a wide range of Ethereum and EVM-compatible networks.
- It includes various utilities for managing wallet connection and credentials, as well as signing transactions on-chain.
// Initialize web3j
Web3j web3 = Web3j.build(new HttpService("https://mainnet.infura.io/v3/your-api-key"));
// Load credentials
Credentials credentials = WalletUtils.loadCredentials("password", "wallet.json");
// Send transaction
TransactionReceipt receipt = Transfer.sendFunds(
web3, credentials,
"0x...", // to address
BigDecimal.valueOf(1.0), // value in ether
Convert.Unit.ETHER // unit
).send();
Web3.py
It is a clone of web3.js but implemented in Python programming language and thus is widely used among python developers. It provides a similar set of functionalities for interacting with EVM-compatible blockchains.
Key Features:
- It provides smooth integration with all Python libraries and frameworks.
- It provides support to Ethereum and EVM Compatible, including smart contract interactions, transactions, and blockchain data access
- Provides a Python interface for working with Ethereum, making it intuitive for developers familiar with the Python ecosystem
- Offers utilities for managing wallets, signing transactions, and interacting with various Ethereum clients
from web3 import Web3
# Initialize Web3
w3 = Web3(Web3.HTTPProvider('https://mainnet.infura.io/v3/your-api-key'))
# Check connection
print(w3.isConnected())
# Get latest block
latest_block = w3.eth.get_block('latest')
print(f"Latest block number: {latest_block['number']}")
Whal3s
Whal3s is a developer platform focused on simplifying the creation, delivery, and management of token utilities. It supports a range of blockchain networks, making it a versatile tool for token-centric projects.
Key Features:
- It focuses on reducing the complexity of token-related development tasks, allowing developers to focus on the implementation of the logic for the application.
- It provides a suite of tools and services from token creation to distribution, and management of assets.
- It supports multiple blockchain networks, including Ethereum, Polygon, and other EVM-compatible chains.
- It offers a flexible and customizable environment for developers to build and deploy token-based applications as per their requirements.
// Initialize Whal3s
const whal3s = new Whal3s('your-api-key');
// Create utility
const utility = await whal3s.createUtility({
name: 'My Token Utility',
chain: 'ethereum',
contractAddress: '0x...'
});
// Verify token ownership
const verification = await utility.verify(walletAddress);
console.log('Token verified:', verification.isValid);
Etherspot TransactionKit
Etherspot TransactionKit is the world’s first React library that simplifies the process of managing transactions across multiple blockchain networks. It aims to provide a seamless and efficient way for React developers to integrate Web3 functionality into their applications.
Key Features:
- It eliminates the low-level complexities of blockchain development.
- It streamlines the handling of transactions, including signing, sending, and monitoring transactions on the network.
- It provides support for multiple blockchain networks, including Ethereum, Polygon, and others
- It provides a very user-friendly, react-based interface for interacting with the blockchain application.
import { TransactionKit } from '@etherspot/transaction-kit';
function App() {
return (
<TransactionKit>
<button onClick={async () => {
const tx = await sendTransaction({
to: '0x...',
value: ethers.utils.parseEther('0.1')
});
console.log('Transaction sent:', tx.hash);
}}>
Send Transaction
</button>
</TransactionKit>
);
}
Moralis Streams API
The Moralis Streams API is a solution for real-time blockchain data integration. It allows developers to seamlessly stream on-chain events directly into their applications, ensuring their dApps stay synchronized with the data on the blockchain in real-time.
Key Features:
- It provides a simple and efficient way to process any event happening on the network.
- It extends support to multiple blockchain networks, including Ethereum, Polygon, and others
- It offers advanced filtering and data processing capabilities to process data in real time.
// Initialize Moralis Streams
const stream = await Moralis.Streams.add({
chains: ["eth"],
description: "Monitor NFT transfers",
tag: "nft",
webhookUrl: "https://your-webhook.com",
abi: [{
"anonymous": false,
"inputs": [
{"indexed": true, "name": "from", "type": "address"},
{"indexed": true, "name": "to", "type": "address"},
{"indexed": true, "name": "tokenId", "type": "uint256"}
],
"name": "Transfer",
"type": "event"
}]
});
// Get stream status
const status = await stream.getStatus();
console.log('Stream status:', status);
Conclusion
With new protocols coming into existence every day, the developers have more and more ecosystems to build on. Web3 libraries are a very important ecosystem tool that offers developers the right set of tools and ease to build an application on a blockchain network. Whether you’re building a simple to-do list dApp or complex DEX or DeFi protocols, the ecosystem has the right set of tools and libraries available for the developers. While you list out the project requirements it is then you need to choose which web3 libraries will be well-suited for the application you are building.
Start building on any ecosystem be it Ethereum, Aptos, or Sui and implement the web3 libraries you just learned about here.