Table of Contents
With the tech industry moving at such a fast pace, it becomes difficult for developers to keep up. New tech stack, new programming language, and new domains keep coming up but rust programming language has been in talks in the tech industry for quite a long time. The awareness of Rust has significantly increased in 2024, it continues to gain momentum as the language of choice by developers for building reliable, efficient, and secure software systems not just in web2 but also web3. Blockchains are coming up with new programming languages that are an optimized version of Rust. From a developer’s point of view learning rust is a valuable skill addition to the portfolio.
Why should developers learn Rust in 2024?
The software industry has witnessed remarkable shifts in recent years, and Rust’s position has strengthened significantly. Major technology companies including Microsoft, Google, and Amazon have not only adopted Rust but are actively investing in its ecosystem. This industry backing translates into growing more and more job opportunities and an amazing community of Rustaceans. According to the Stack Overflow Developer Survey, Rust has maintained its position as the most loved programming language for the ninth consecutive year, with an unprecedented 87% developer satisfaction rate.
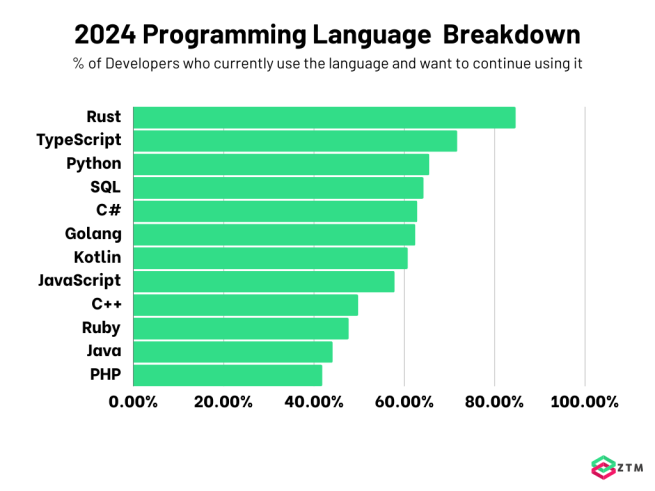
Features of Rust
1. High Performance Rust delivers C/C++-level performance with a more approachable syntax. It excels in performance-critical applications like operating systems, embedded systems, and game engines, offering low-level control without sacrificing code readability.
2. Memory Safety Without Garbage Collection Unlike other high-level languages, Rust manages memory without a garbage collector through its ownership and borrow-checking system. This ensures memory safety at compile-time, eliminating common issues like memory leaks and data races while maintaining optimal performance.
3. Concurrency Without Fear Rust’s compiler enforces safe concurrent programming by design. The ownership model prevents data races at compile-time, making it significantly easier to write reliable multi-threaded applications without the typical headaches of concurrent programming.
4. Strong Type System and Compiler The Rust compiler acts as a strict but helpful guardian, catching potential issues before runtime. While its strictness might challenge beginners, it ultimately leads to more robust and reliable code by preventing common programming mistakes during development rather than in production.
5. Developer-Friendly and Community-Supported Despite its learning curve, Rust offers excellent documentation, tutorials, and community support. The package manager, Cargo, streamlines dependency management and project setup, making it easier to maintain and scale projects effectively.
6. Versatile and Growing Ecosystem Rust supports diverse development needs, from web frameworks like Rocket and Actix to systems programming and blockchain applications. Major companies including Mozilla, Microsoft, and Facebook have adopted Rust, contributing to its growing ecosystem of tools and libraries.
7. High Industry Demand As companies prioritize performance and reliability, demand for Rust developers continues to grow. Opportunities span systems programming, cybersecurity, DevOps, and blockchain development, making Rust’s expertise increasingly valuable in the job market.
Learning Roadmap for 2024
The journey to mastering Rust can be broken down into three distinct phases, each building upon the previous one. Your first steps begin with the foundation phase, typically spanning two to four weeks. During this period, you’ll set up your development environment, including the Rust toolchain and your preferred IDE. You’ll begin writing your first programs using Cargo, Rust’s powerful package manager while learning the basic syntax and fundamental programming constructs.
As you progress through the foundation phase, you’ll encounter Rust’s most distinctive feature: its ownership system. This concept, while initially challenging, forms the cornerstone of Rust’s memory safety guarantees. You’ll learn how variables own their data, how to share data through borrowing, and how the compiler ensures memory safety through lifetime tracking. Along with these core concepts, you’ll work with Rust’s basic collections and types, gradually building a solid understanding of how Rust programs are structured.
The intermediate phase, typically lasting four to six weeks, delves deeper into Rust’s sophisticated type system. Here you’ll master traits and implementations, understanding how Rust achieves abstraction and code reuse without traditional inheritance. Generic programming becomes a powerful tool in your arsenal, allowing you to write flexible, reusable code while maintaining type safety. This phase also introduces you to Rust’s approach to error handling and memory management patterns that go beyond the basics.
Advanced development marks the final phase of your initial learning journey, usually spanning six to eight weeks. During this time, you’ll explore Rust’s powerful concurrency features, including async programming and thread safety mechanisms. You’ll learn to leverage the ecosystem’s most popular crates and frameworks while developing real-world applications. This phase transforms you from a Rust learner into a Rust developer, capable of building production-ready software.
Step 1: Fundamentals
- Read “The Rust Programming Language” book
- Complete “Rustlings” exercises
- Master basic concepts:
- Variables and mutability
- Data types
- Functions
- Control flow
- Ownership and borrowing
Step 2: Intermediate Concepts
- Understand the module system
- Learn about error handling
- Practice with generics and traits
- Study collections and smart pointers
- Explore concurrent programming
Step 3: Advanced Topics
- 1. Foreign Function Interface (FFI)
- 2. Unsafe Rust
- 3. Advanced traits
- 4. Advanced types
- 5. Macros
How to Learn Rust
Getting Started
Beginning your Rust journey starts with setting up the development environment. On Unix-like systems, installation is straightforward through the rustup tool:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Windows users can download the rustup-init.exe installer from rustup.rs
. The installation provides essential tools including rustup for version management, cargo for package management and building, rustfmt for code formatting, and clippy for advanced linting.
Project Ideas to build
The best way to learn rust is to build more and more projects to practice the concepts. Let us look at a few project ideas to build while you learn rust to bring your knowledge into practice.
Beginner Level Projects
Command-Line File Analyzer – Build a tool that analyzes files in a directory. This project covers concepts like file I/O, error handling, basic data structures, and string manipulation techniques.
Personal Task Manager – Build a CLI task manager that stores tasks in a JSON file. This project will cover concepts like serialization/deserialization with serde, basic CRUD operations, enum types and pattern matching.
Memory Game – Build a terminal-based memory-matching game. This project covers concepts like random number generation, terminal manipulation with cross term or termion, and state management. It also talks about the ownership system while handling game state and user input.
Intermediate Level Projects
HTTP Load Tester – Build a concurrent HTTP load testing tool. This project introduces async programming with Tokio, HTTP clients, and concurrent data collection. You’ll learn about Rust’s async/await syntax, channels for communication between threads, and handling multiple concurrent connections.
Custom Database– Build a simple key-value store with persistence. This project will cover concepts like file I/O, data structures, and binary serialization. You can start with basic CRUD operations, then add features like supporting transactions, write-ahead logging, basic query language, and data compression This project will let you master concepts like Rust’s traits system, error handling, and performance optimization.
Cross-Platform GUI File Browser – Create a file browser using a GUI framework like egui or iced. This project covers important concepts like event handling and state management. It will also cover Rust’s platform-specific features and how to maintain a clean architecture and implement GUI programming in Rust, perform cross-platform file operations, and asynchronous operations in GUI applications.
Sneak peek into Rust code
Let us quickly look at a Hello World code in Rust so that you can get an idea of how to start and you can hop on to your learning Rust journey now! Our code has three main points to pay attention to, the fn
keyword, main()
, and println!
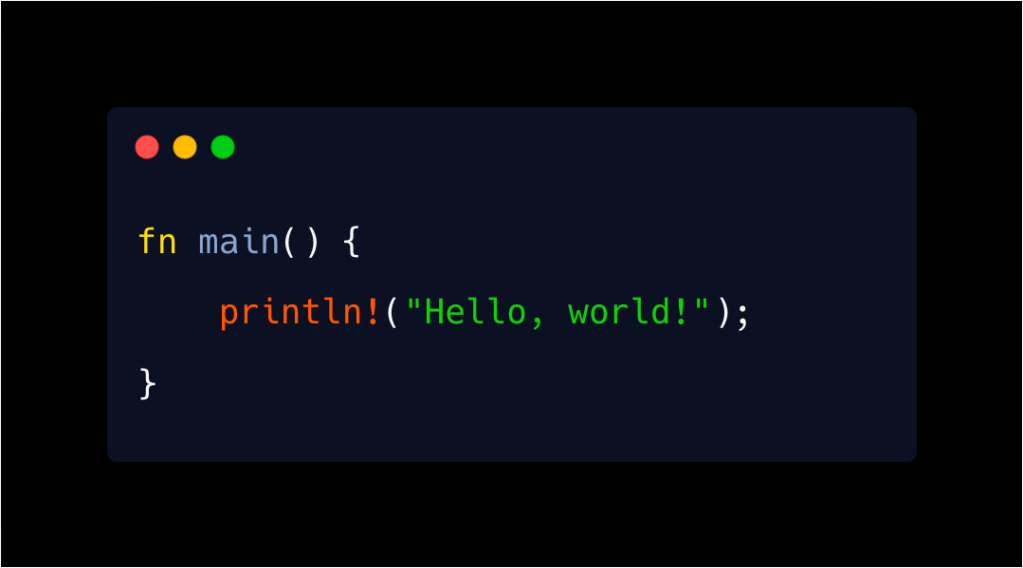
1. fn main()
This is our main function, and it’s super important in Rust. Here’s why:
fn
tells Rust we’re declaring a functionmain
is a special name – it’s the entry point of every Rust program- The empty parentheses
()
mean this function doesn’t take any parameters - Your program must have a main function, or it won’t run. It’s that simple!
2. println!
This is what we call a macro in Rust, and you can spot it by the exclamation mark:
- It’s not a regular function (that’s why it has the
!
) - Macros are like code generators — they expand into more code during compilation
println!
specifically handles printing text to the console- The
!
isn’t just for show – it’s how Rust distinguishes macros from regular functions
Now how do we run this code? You can either use the RustRover IDE online or run the file locally through your terminal or VS Code. To run locally, open the terminal and run the ./main command. Your output should be as shown below:

Build on MANTRA using your Rust skills
Mantra Chain uses pure rust as its smart contract language. The Introduction to MANTRA Chain covers more about the Cosmos ecosystem, RWA’s, Architecture of the Chain, and so on. The Building on Mantra Chain covers pure Rust concepts like data types, operators, strings, function modules everything that you would anyway cover while you learn Rust so make use of those Rust skills and build some cool dApps on Mantra Chain.
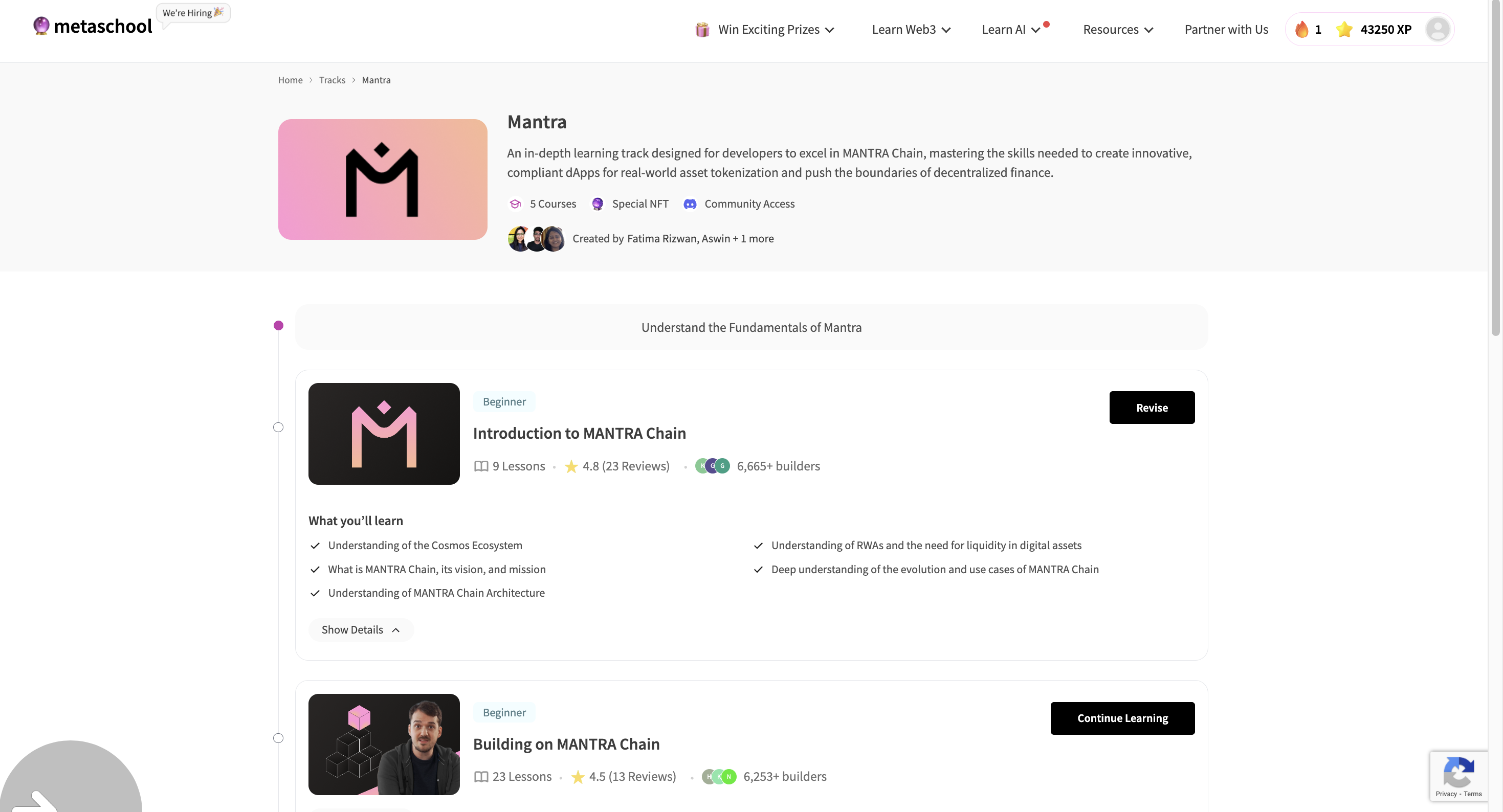
Conclusion
Learning Rust in 2024 is more of an investment than just adding another programming language to your toolkit. It introduces you to a new way of thinking about software development, where the compiler becomes a helpful partner in writing correct, efficient code. While the learning curve may be steep, particularly around the complicated concepts of ownership system, the benefits of memory safety, performance, and more this language is a goldmine not just for web2 developers but also for web3 developers.
Blockchain ecosystems like Fuel, Aptos, Sui, and MANTRA all use rust in one way or the other as their smart contract language. While you are on your learning journey don’t forget to refer to our MANTRA Chain courses to use your RUST skills and build a dApp on-chain.
Frequently Asked Questions(FAQ’s)
Is learning Rust worth it in 2024?
Mastering any programming language takes time and so does Rust, but the payoff is worth it. It is already a widely used language in web3 as well as web2 development which opens a lot of opportunities for developers learning this language. Stick with it; you’ll find that Rust can be fun and rewarding.
Is Rust harder to learn than Python ?
Rust is slightly more complex than other languages, meaning it has a relatively higher learning curve compared to Python.
What are the few use cases of Rust?
Rust is widely used in web development, with a lot of blockchains using it for smart contract programming. It also finds a wide number of use cases in the fields of IoT, Gaming, Embedded Systems, etc.