Table of Contents
The switch case statement simplifies decision-making in programming by offering a more organized way to handle multiple conditions compared to Python’s if
, elif
, and else
structure.
In Python, the if
statement runs code if a condition is true, otherwise it runs code under else
. Multiple conditions can be handled with elif
statements. However, this approach can become cumbersome with numerous elif
conditions and indentation levels. This is where the switch case statement provides a cleaner alternative.
What is a Switch Case Statement?
Switch case statements, found in many other programming languages, offer a more structured way to handle scenarios where multiple comparisons are required to make a decision. They allow us to branch execution to different parts of the code based on the value of an expression, providing a cleaner alternative to lengthy if-elif-else
chains.
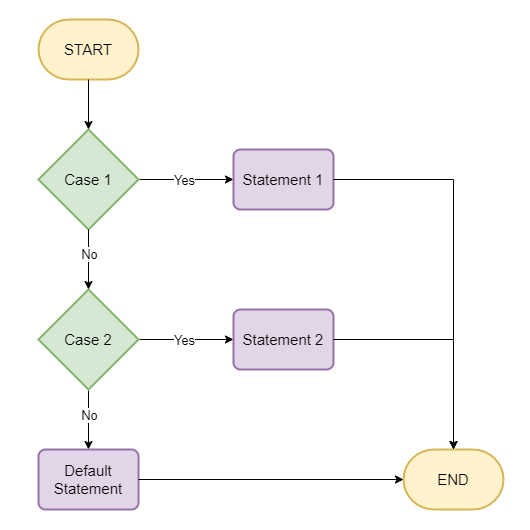
The flow chart on the left illustrates the workings of a switch case statement.
- The switch case expression is evaluated once.
- The value of the evaluated expression is compared with each case.
- If a match is found the corresponding statement (or block of code) is executed – End Switch.
- If no match is found, the default statement gets executed – End Switch.
Switch Case is a selection control statement. Also known as a conditional control statement, these statements direct the flow of execution based on whether certain conditions are true or false. It evaluates conditions and executes different blocks of code depending on the outcome.
Although Python lacks traditional switch case statements, it introduces similar functionality with the match
and case
statements starting from Python 3.10. Let’s learn about it in more detail.
Python’s Match and Case
Syntax
1. match
Statement:
The match
statement is used to start pattern matching on a variable. It examines the value of the variable against various patterns defined in the subsequent case
statements.
match variable:
# Pattern matching cases here
2. case
Statement:
Each case
specifies a pattern to match against the variable’s value. The code block following the case
is executed if the pattern matches. You can use guards (additional conditions) with case
statements to refine the matching criteria.
match variable:
case pattern:
# Code to execute if pattern matches
3. Using Guards:
Guards are conditional expressions that can be used in case
statements to add extra conditions to the match.
match variable:
case _ if condition:
# Code to execute if condition is true
The underscore can be used for two purposes:
- Wildcard Pattern: The underscore
_
is used as a wildcard pattern that matches any value. It acts as a catch-all for values that do not match other specific patterns defined in thecase
statements.
2. Guard Conditions: When used with a guard (if condition
), the underscore in case _ if condition:
means that any value is acceptable as long as the condition
specified in the guard is true. This allows additional conditions to be applied to the match process.
Example
def evaluate_number(n):
match n:
case 1:
print("Number is one.")
case 2:
print("Number is two.")
case _ if n < 0:
print("Number is negative.")
case _ if n % 2 == 0:
print("Number is even.")
case _:
print("Number is odd and positive.")
evaluate_number(4) # Output: Number is even.
case 1:
Matches ifn
is exactly.case 2:
Matches ifn
is exactly.case _ if n < 0:
Matches any value ofn
if it is less than0
. Here,_
indicates that the specific value is not important as long as the conditionn < 0
is true.case _ if n % 2 == 0:
Matches any value ofn
if it is an even number. The underscore_
is used here to indicate that any value can be matched as long as it satisfies the conditionn % 2 == 0
.case _:
Acts as a default case that catches any value not matched by the previouscase
statements.
Code Implementation
The match
statement allows for pattern matching, where you can check a variable against multiple patterns. Each case
within the match
block specifies a pattern to compare with the variable’s value, enabling clear and concise branching logic.
This concept can be more clearly illustrated with an example that converts a basic if
, elif
, and else
structure into a match
and case
structure.
def determine_age_group(age):
if age < 0:
return "Invalid age"
elif age <= 12:
return "Child"
elif age <= 19:
return "Teenager"
elif age <= 64:
return "Adult"
else:
return "Senior"
print(determine_age_group(25)) # Output: Adult
Explanation: The determine_age_group()
function categorizes a person’s age into life stages. It checks if the age is invalid (less than 0), or falls into specific ranges: “Child” (0-12), “Teenager” (13-19), “Adult” (20-64), or “Senior” (65 and older). For the input 25, it matches the “Adult” category and returns “Adult”, which is then printed.
In the match
–case
version, we use pattern matching with guard conditions (using if
within the case
statements) to handle the different age ranges and special cases. This provides a similar logical structure to the if
, elif
, and else
approach but leverages the new pattern matching capabilities introduced in Python 3.10.
def determine_age_group(age):
match age:
case _ if age < 0:
return "Invalid age"
case age if age <= 12:
return "Child"
case age if age <= 19:
return "Teenager"
case age if age <= 64:
return "Adult"
case _:
return "Senior"
print(determine_age_group(25)) # Output: Adult
Explanation: The determine_age_group()
function utilizes Python’s match
and case
statements to categorize a person’s age.
- It begins with the
match age:
statement, which initiates the pattern matching process forage
. - The function then evaluates a series of
case
statements. If the case is true, the subsequent code block, a return statement in this example, is executed. - The cases match ages falling within specific ranges:
age<0
for “Invalid age”,age<=12
for “Child”,age<=19
for “Teenager”, andage<=64
for “Adult”. The final case,case _:
, acts as a default catch-all for ages above 64, returning “Senior”. - When
determine_age_group(25)
is called, the function matches theage<=64
case, thus returning “Adult”, which is then printed.
Conclusion
Python uses match
and case
statements to imitate the functionality of Switch Case. It offers a modern, organized alternative to if
, elif
, and else
for handling multiple conditions. This approach simplifies complex decision-making and improves code readability, making it a valuable tool for Python developers.
Python is also crucial in the context of blockchain development. Its versatility and powerful libraries make it a good choice for building and interacting with blockchain systems. To understand why Python is so effective for blockchain projects, check out our article, 7 Reasons Why You Should Develop a Blockchain Using Python which explores the numerous advantages of using Python for blockchain development.