Table of Contents
Whether you’re reading data, writing information, or performing file manipulations, one common use case is needing to check if a file exists before attempting to access it for reading, writing, or appending. This is a crucial step to avoid errors and ensure that the code runs smoothly. If you attempt to perform any file operations and the file doesn’t exist, your program might end up crashing. Fortunately, Python provides straightforward methods to determine the presence of a file.
In this article, we will discuss the different methods for checking if a file exists in Python. Whether you are working on a small script or a larger application, mastering this skill is essential for robust file handling in your projects.
Familiarisation with the File Structure
It is important to understand how to inspect and navigate through your working directory structure is essential for effective file management in Python. We can use two methods to observe the contents of the directories.
- Get Your Current Directory Using
os.getcwd()
: To find out your current working directory in Python, you can utilize thegetcwd()
function from theos
module. This function returns the path of the current working directory as a string.
Let’s understand how it works using code implementation:
import os
# Get the current working directory
current_directory = os.getcwd()
print("The current working directory is:", current_directory)
The output of the code can be observed in the screenshot below.
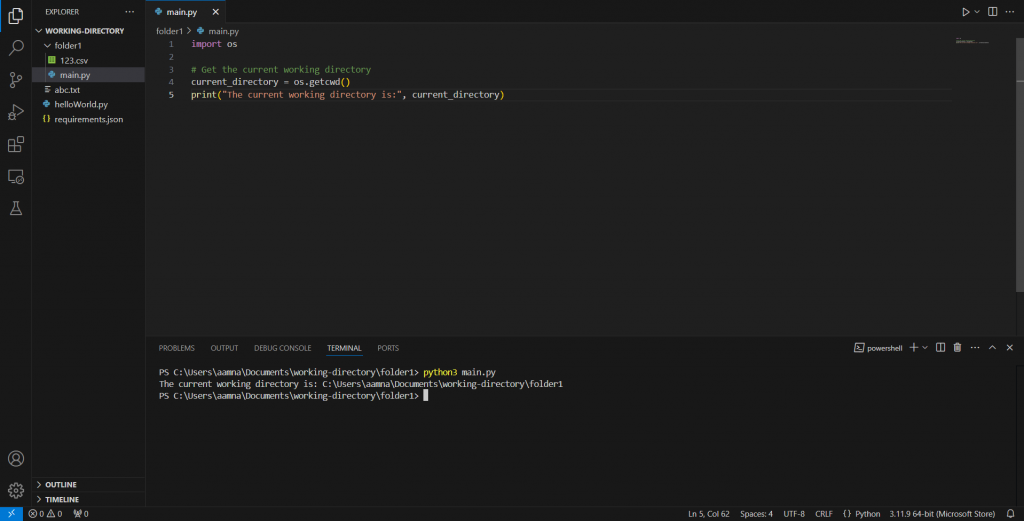
- List All Files and Folders in Your Directory Using
os.listdir()
: To view all the files and folders in the current directory, thelistdir()
function from theos
module comes in handy. This function returns a list of the names of entries in the specified directory.
import os
# Get the current working directory
current_directory = os.getcwd()
# List all files and folders in the current directory
entries = os.listdir(current_directory)
print(entries)
The output of the code can be observed in the screenshot below.
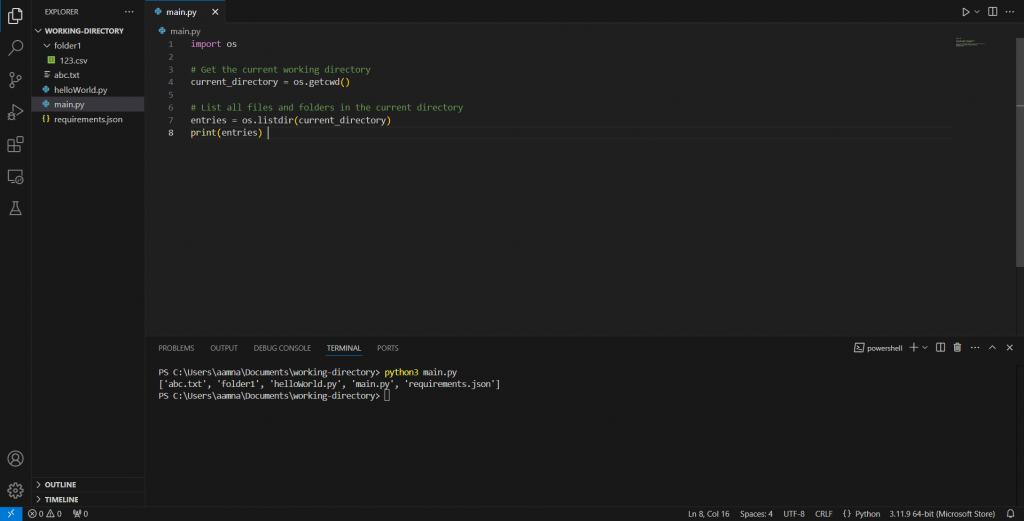
How to check if the file exists?
Having understood how to navigate directories, let’s move on to checking if specific files exist.
Method 1: os.path.exists()
The os.path.exists()
function from the os
module provides a straightforward way to determine whether a file or directory exists.
import os
# Define the path to the file
file_path = '<folder-name>/<file-name>'
# Check if the file exists
if os.path.exists(file_path):
print("The file exists.")
else:
print("The file does not exist.")
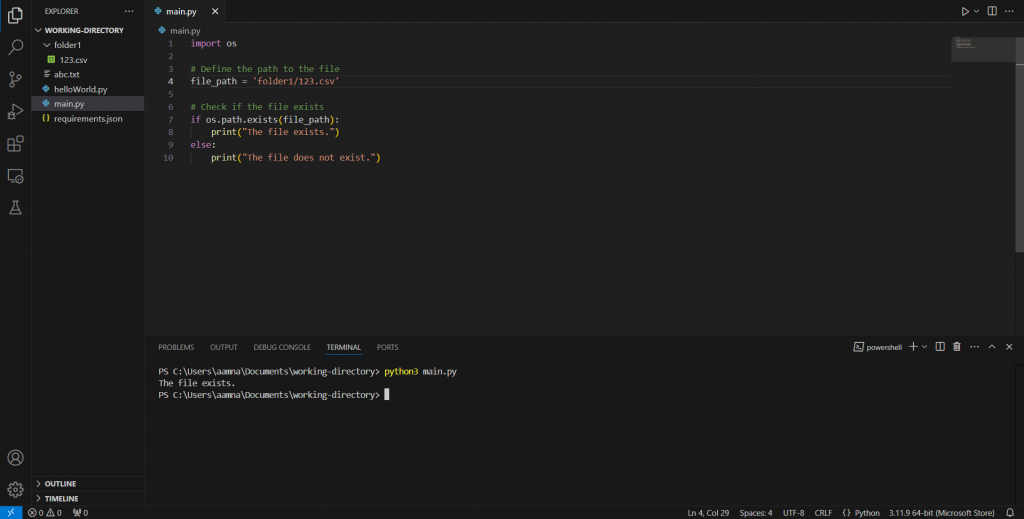
Explanation:
file_path
specifies the location of the file relative to the current working directory.os.path.exists(file_path)
returnsTrue
if the file or directory exists at the specified path, andFalse
otherwise.- The
if
statement checks the result and prints a message based on whether the file is present.
Method 2: pathlib.Path.exists()
For a more modern, object-oriented approach, you can use the pathlib
module’s Path.exists()
method.
from pathlib import Path
# Create a Path object
file_path = Path('<folder-name>/<file-name>')
# Check if the file exists
if file_path.exists():
print("The file exists.")
else:
print("The file does not exist.")
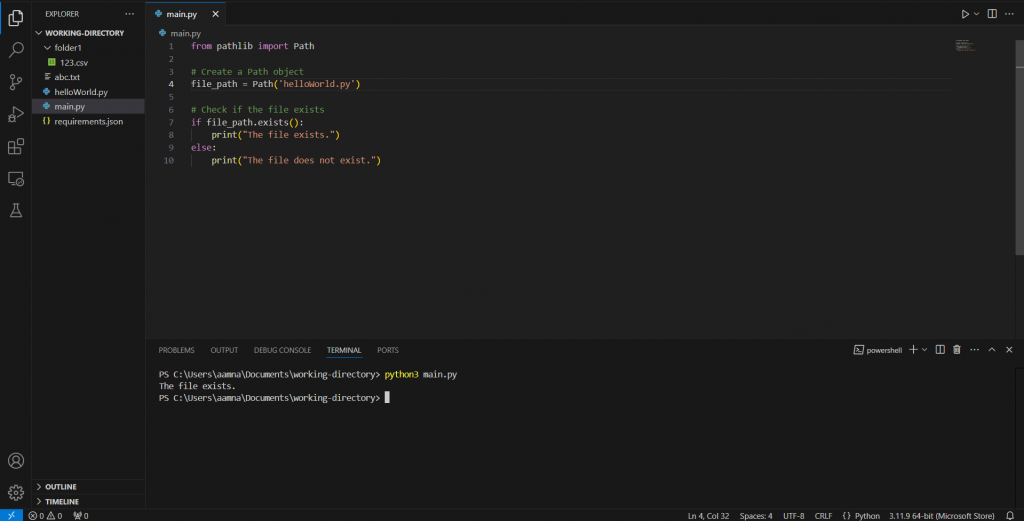
Explanation:
Path('<folder-name>/<file-name>')
creates aPath
object representing the file path.file_path.exists()
checks if the file or directory exists at that path and returnsTrue
orFalse
.- The
if
statement evaluates the result and prints the appropriate message based on the file’s presence.
Method 3: Try-Except Block with open()
Another approach is to use a try-except block with the open()
function. This method not only checks if the file exists but also attempts to open it, combining existence checks with file access.
try:
# Attempt to open the file
with open('<folder-name>/<file-name>', 'r') as file:
print("The file exists.")
except FileNotFoundError:
print("The file does not exist.")
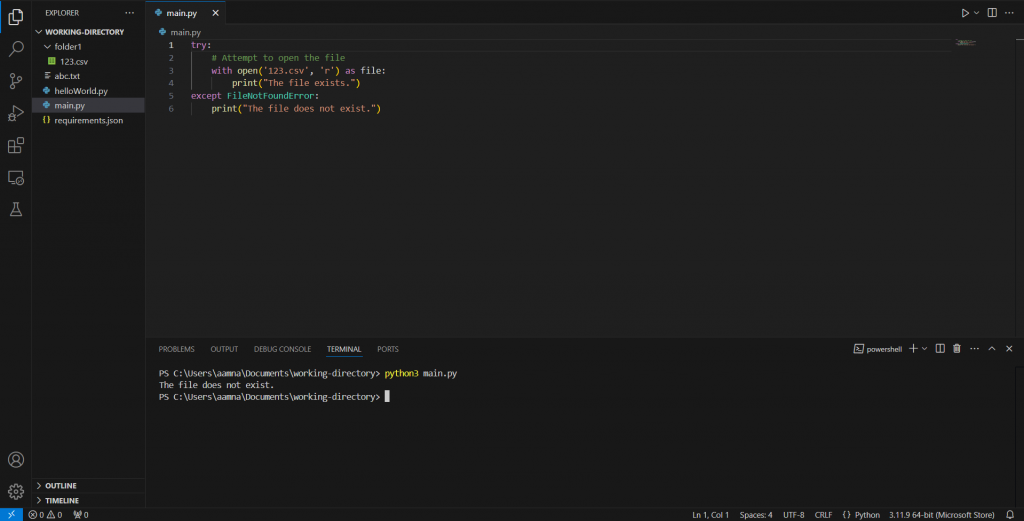
Explanation:
- The
try
block attempts to open the file in read mode ('r'
). - If the file is found, the
with
statement allows you to interact with the file, and the message “The file exists.” is printed. - If the file is not found, a
FileNotFoundError
is raised, and theexcept
block catches this error and prints “The file does not exist.”
Each of these methods provides a reliable way to check for the existence of a file, so you can choose the one that best fits your needs and coding style.
Conclusion
Verifying the existence of a file is a crucial step in file handling and can be approached in several effective ways using Python. We have discussed three methods of checking if a file exists in the desired location:
os.path.exists()
pathlib.Path.exists()
- Try-Except Block with
open()
By understanding and implementing these methods, you can avoid errors related to file operations and enhance the overall functionality of your applications. Choose the method that best suits your needs and coding style, and you’ll be well-equipped to manage files effectively in your Python projects.
Python is also crucial in the context of blockchain development. Its versatility and powerful libraries make it a good choice for building and interacting with blockchain systems. To understand why Python is so effective for blockchain projects, check out our article, 7 Reasons Why You Should Develop a Blockchain Using Python which explores the numerous advantages of using Python for blockchain development.
FAQs
What is the purpose of checking if a file exists in Python?
Checking if a file exists helps prevent errors in your code by ensuring that operations like reading from or writing to a file are only performed if the file is actually present. This can avoid exceptions and improve the robustness of your program.
What is the difference between os.path.exists()
and pathlib.Path.exists()
?
os.path.exists()
is a function from the os
module that checks if a file or directory exists at a given path. pathlib.Path.exists()
is a method from the pathlib
module, which provides a more modern, object-oriented way to handle filesystem paths. Both accomplish the same task, but pathlib
offers a more intuitive interface and additional functionality for path manipulations.
Can I check if a file exists using a try-except block?
Yes, you can use a try-except block by attempting to open the file. If the file does not exist, a FileNotFoundError
exception will be raised. This method is useful if you also need to handle file operations in addition to checking existence.
try:
with open(‘path/to/file.txt’, ‘r’) as file:
print(“The file exists.”)
except FileNotFoundError:
print(“The file does not exist.”)