Table of Contents
Introduction
Decentralized applications (dApps) represent the cornerstone of Web3 technology, combining traditional web development with blockchain capabilities. Unlike conventional applications, dApps operate on a decentralized network—specifically, the Ethereum blockchain—providing transparency, immutability, and trustless execution of business logic through smart contracts. This comprehensive guide will walk you through building a full-stack Ethereum dApp, from smart contract development to frontend integration and deployment.
Prerequisites
Before diving into development, ensure you have the following tools and knowledge:
Development Tools
- Node.js (v14.0.0 or later)
- npm or yarn package manager
- Metamask browser extension
- Code editor (VS Code recommended with Solidity extensions)
- Git for version control
Technical Knowledge
- JavaScript/TypeScript fundamentals
- React.js framework basics
- Solidity programming language
- Basic understanding of blockchain concepts
- Familiarity with Web3 principles
Resources
- Some ETH in your test wallet (using Sepolia other testnet faucets)
- Infura or Alchemy account for API access
- GitHub account for version control
1. Setting Up the Development Environment
Initial Setup
First, let’s create a robust development environment with all the necessary tools and configurations:
# Create project directory
mkdir ethereum-dapp
cd ethereum-dapp
# Initialize npm project
npm init -y
# Install core dependencies
npm install --save-dev hardhat @nomiclabs/hardhat-waffle ethereum-waffle chai @nomiclabs/hardhat-ethers ethers @openzeppelin/contracts dotenv
# Install development tools
npm install --save-dev prettier prettier-plugin-solidity solhint
# Initialize Hardhat project
npx hardhat init
Project Structure
Create a well-organized project structure for your dApp:
ethereum-dapp/
├── contracts/
├── scripts/
├── test/
├── frontend/
├── hardhat.config.js
├── .env
└── .gitignore
Configuration Setup
Create a Hardhat configuration file for your dApp:
require("@nomiclabs/hardhat-waffle");
require("@nomiclabs/hardhat-ethers");
require("dotenv").config();
const INFURA_PROJECT_ID = process.env.INFURA_PROJECT_ID;
const PRIVATE_KEY = process.env.PRIVATE_KEY;
module.exports = {
solidity: {
version: "0.8.19",
settings: {
optimizer: {
enabled: true,
runs: 200
}
}
},
networks: {
hardhat: {
chainId: 1337
},
sepolia: {
url: `https://sepolia.infura.io/v3/${INFURA_PROJECT_ID}`,
accounts: [`0x${PRIVATE_KEY}`],
gas: 2100000,
gasPrice: 8000000000
},
mainnet: {
url: `https://mainnet.infura.io/v3/${INFURA_PROJECT_ID}`,
accounts: [`0x${PRIVATE_KEY}`]
}
},
paths: {
sources: "./contracts",
tests: "./test",
cache: "./cache",
artifacts: "./frontend/src/artifacts"
}
};
2. Writing the Smart Contract
Contract Development
Our smart contract is a simple storage system that lets users store and retrieve values but with important safety features added. We use OpenZeppelin’s contracts to handle basic security (like preventing reentrancy attacks) and ownership controls. The contract stores a value for each user’s address separately, tracks who has stored values, and lets the owner perform bulk operations if needed. When values change, the contract emits events so the value on the frontend of our dApp can update in real time.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
import "@openzeppelin/contracts/access/Ownable.sol";
import "@openzeppelin/contracts/security/ReentrancyGuard.sol";
contract SimpleStorage is Ownable, ReentrancyGuard {
// State variables
uint256 private value;
mapping(address => uint256) private userValues;
mapping(address => bool) private hasStored;
// Events
event ValueChanged(address indexed user, uint256 newValue, uint256 timestamp);
event ValueReset(address indexed user, uint256 timestamp);
// Custom errors
error InvalidValue();
error NoValueStored();
// Modifiers
modifier validValue(uint256 _value) {
if (_value == 0) revert InvalidValue();
_;
}
// Main functions
function setValue(uint256 newValue) public validValue(newValue) nonReentrant {
value = newValue;
userValues[msg.sender] = newValue;
hasStored[msg.sender] = true;
emit ValueChanged(msg.sender, newValue, block.timestamp);
}
function getValue() public view returns (uint256) {
return value;
}
function getUserValue(address user) public view returns (uint256) {
if (!hasStored[user]) revert NoValueStored();
return userValues[user];
}
function resetValue() public onlyOwner {
value = 0;
emit ValueReset(msg.sender, block.timestamp);
}
// Batch operations
function setMultipleValues(address[] calldata users, uint256[] calldata values)
public
onlyOwner
{
require(users.length == values.length, "Arrays length mismatch");
for (uint i = 0; i < users.length; i++) {
userValues[users[i]] = values[i];
hasStored[users[i]] = true;
emit ValueChanged(users[i], values[i], block.timestamp);
}
}
}
3. Testing the Smart Contract
The test file checks if our contract works as intended by simulating real user interactions. We test three main things: that the contract deploys correctly with the right owner, that users can store and retrieve their values properly, and that only the owner can perform restricted operations like bulk updates. Each test uses different test accounts to simulate multiple users interacting with the contract.
const { expect } = require("chai");
const { ethers } = require("hardhat");
describe("SimpleStorage", function() {
let SimpleStorage;
let simpleStorage;
let owner;
let addr1;
let addr2;
beforeEach(async function() {
SimpleStorage = await ethers.getContractFactory("SimpleStorage");
[owner, addr1, addr2] = await ethers.getSigners();
simpleStorage = await SimpleStorage.deploy();
await simpleStorage.deployed();
});
describe("Deployment", function() {
it("Should set the right owner", async function() {
expect(await simpleStorage.owner()).to.equal(owner.address);
});
it("Should initialize with zero value", async function() {
expect(await simpleStorage.getValue()).to.equal(0);
});
});
describe("Transactions", function() {
it("Should set the value correctly", async function() {
await simpleStorage.connect(addr1).setValue(42);
expect(await simpleStorage.getValue()).to.equal(42);
expect(await simpleStorage.getUserValue(addr1.address)).to.equal(42);
});
it("Should emit ValueChanged event", async function() {
await expect(simpleStorage.connect(addr1).setValue(42))
.to.emit(simpleStorage, "ValueChanged")
.withArgs(addr1.address, 42, await getBlockTimestamp());
});
it("Should revert with InvalidValue for zero input", async function() {
await expect(simpleStorage.setValue(0))
.to.be.revertedWithCustomError(simpleStorage, "InvalidValue");
});
});
describe("Batch Operations", function() {
it("Should set multiple values correctly", async function() {
const users = [addr1.address, addr2.address];
const values = [42, 84];
await simpleStorage.setMultipleValues(users, values);
expect(await simpleStorage.getUserValue(addr1.address)).to.equal(42);
expect(await simpleStorage.getUserValue(addr2.address)).to.equal(84);
});
});
});
async function getBlockTimestamp() {
const blockNumBefore = await ethers.provider.getBlockNumber();
const blockBefore = await ethers.provider.getBlock(blockNumBefore);
return blockBefore.timestamp;
}
4. Frontend Development
React Setup with Web3 Integration
Create a new React application with advanced Web3 integration for the frontend of the dApp. The useWeb3Contract hook handles all our blockchain interactions in one place. It connects to MetaMask, creates the contract instance, and provides functions our components can use to interact with the blockchain. When something goes wrong (like MetaMask not being installed or a transaction failing), it captures the error so we can show it to the user. This keeps all the complex blockchain logic separate from our UI code.
// src/hooks/useWeb3Contract.js
import { useState, useEffect, useCallback } from 'react';
import { ethers } from 'ethers';
import SimpleStorage from '../artifacts/contracts/SimpleStorage.sol/SimpleStorage.json';
export function useWeb3Contract(contractAddress) {
const [contract, setContract] = useState(null);
const [provider, setProvider] = useState(null);
const [signer, setSigner] = useState(null);
const [error, setError] = useState(null);
const [loading, setLoading] = useState(true);
const initialize = useCallback(async () => {
try {
if (!window.ethereum) {
throw new Error("MetaMask not installed");
}
const provider = new ethers.providers.Web3Provider(window.ethereum);
const signer = provider.getSigner();
const contract = new ethers.Contract(
contractAddress,
SimpleStorage.abi,
signer
);
setProvider(provider);
setSigner(signer);
setContract(contract);
setError(null);
} catch (err) {
setError(err.message);
} finally {
setLoading(false);
}
}, [contractAddress]);
useEffect(() => {
initialize();
}, [initialize]);
const connectWallet = async () => {
try {
await window.ethereum.request({ method: 'eth_requestAccounts' });
await initialize();
} catch (err) {
setError(err.message);
}
};
return { contract, provider, signer, error, loading, connectWallet };
}
Creating a User Interface Component of the dApp
The StorageInterface component is the main screen with which users interact with the dApp. It shows the currently stored value and has a form where users can input new values. When users submit the form, it calls our smart contract through the Web3 hook we created. While the transaction is processing, it shows a loading state, and if anything goes wrong, it displays an error message. It also handles connecting to MetaMask if users haven’t done that yet.
// src/components/StorageInterface.js
import React, { useState, useEffect } from 'react';
import { useWeb3Contract } from '../hooks/useWeb3Contract';
const CONTRACT_ADDRESS = process.env.REACT_APP_CONTRACT_ADDRESS;
function StorageInterface() {
const [value, setValue] = useState('');
const [storedValue, setStoredValue] = useState(null);
const [isLoading, setIsLoading] = useState(false);
const { contract, error, loading, connectWallet } = useWeb3Contract(CONTRACT_ADDRESS);
useEffect(() => {
if (contract) {
fetchStoredValue();
}
}, [contract]);
const fetchStoredValue = async () => {
try {
const value = await contract.getValue();
setStoredValue(value.toString());
} catch (err) {
console.error('Error fetching value:', err);
}
};
const handleSetValue = async (e) => {
e.preventDefault();
setIsLoading(true);
try {
const tx = await contract.setValue(value);
await tx.wait();
await fetchStoredValue();
setValue('');
} catch (err) {
console.error('Error setting value:', err);
} finally {
setIsLoading(false);
}
};
if (loading) return <div>Loading...</div>;
if (error) return (
<div>
<p>Error: {error}</p>
<button onClick={connectWallet}>Connect Wallet</button>
</div>
);
return (
<div>
<h2>Storage Interface</h2>
<p>Current Value: {storedValue ?? 'No value stored'}</p>
<form onSubmit={handleSetValue}>
<input
type="number"
value={value}
onChange={(e) => setValue(e.target.value)}
placeholder="Enter a value"
disabled={isLoading}
/>
<button type="submit" disabled={isLoading || !value}>
{isLoading ? 'Processing...' : 'Set Value'}
</button>
</form>
</div>
);
}
export default StorageInterface;
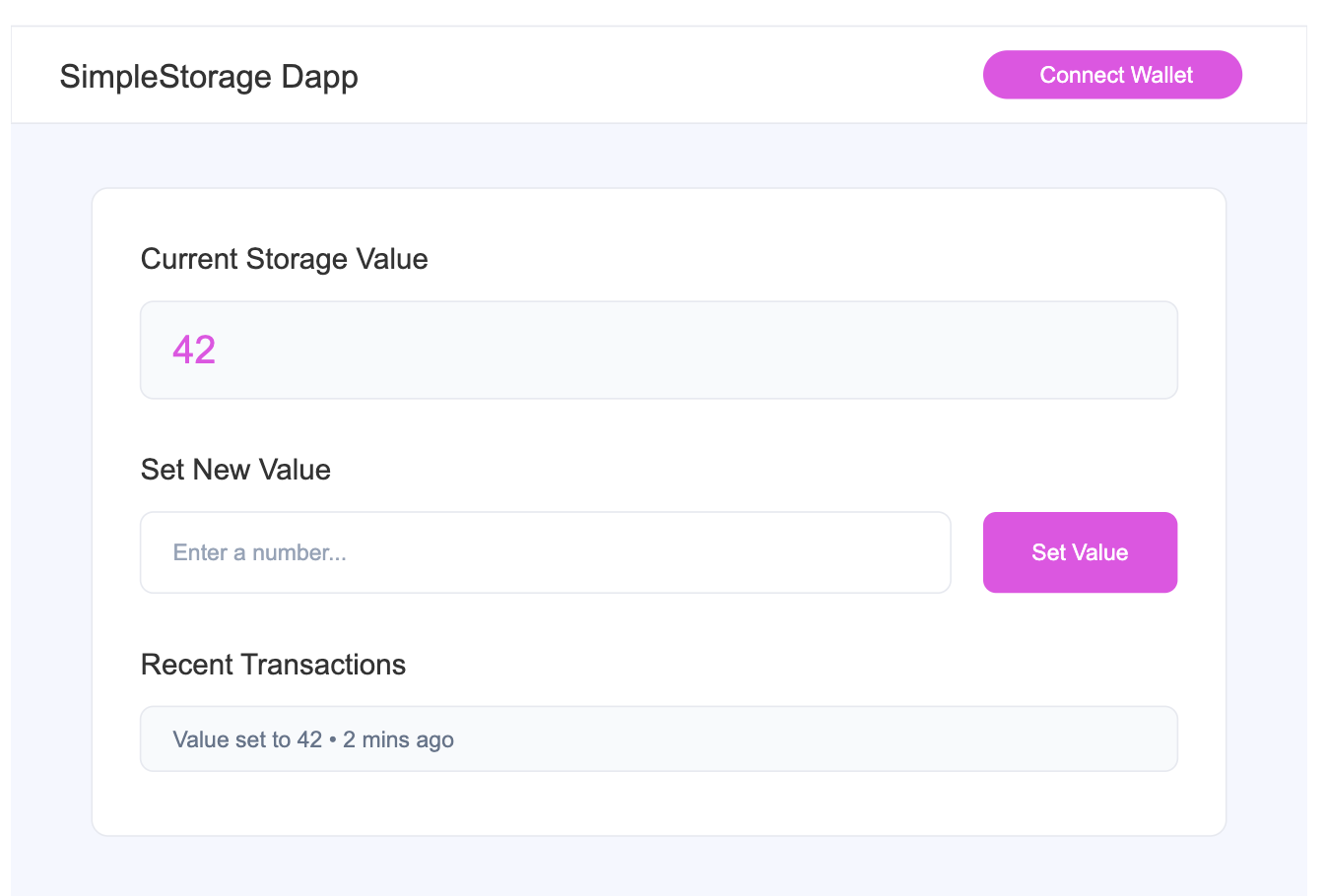
5. Deployment and Production
Smart Contract Deployment
Create a deployment script with proper error handling and verification. Our deployment script handles the process of putting the smart contract on the blockchain. It first checks if we have enough ETH to deploy, then deploys the contract and waits for it to be confirmed. If we’re on a testnet, it also verifies the contract on Etherscan so users can read the source code. The script provides clear console messages about what’s happening during deployment.
// scripts/deploy.js
async function main() {
try {
// Get network information
const [deployer] = await ethers.getSigners();
console.log("Deploying contracts with account:", deployer.address);
const balance = await deployer.getBalance();
console.log("Account balance:", ethers.utils.formatEther(balance));
// Deploy contract
const SimpleStorage = await ethers.getContractFactory("SimpleStorage");
const simpleStorage = await SimpleStorage.deploy();
await simpleStorage.deployed();
console.log("SimpleStorage deployed to:", simpleStorage.address);
// Verify contract on Etherscan
if (network.name !== "hardhat") {
console.log("Waiting for block confirmations...");
await simpleStorage.deployTransaction.wait(6);
await hre.run("verify:verify", {
address: simpleStorage.address,
constructorArguments: [],
});
}
} catch (error) {
console.error("Deployment failed:", error);
process.exit(1);
}
}
main()
.then(() => process.exit(0))
.catch((error) => {
console.error(error);
process.exit(1);
});
Best Practices for Ethereum Development
When developing on the Ethereum blockchain, following established best practices is crucial for creating secure and efficient applications. Start by ensuring you’re using the latest stable version of Solidity, as each new release brings important security patches and features that can enhance your smart contracts. Security should be at the forefront of your development process – implement proper access controls, use tested design patterns, and consider having your contracts audited before deployment to mainnet.
Gas optimization is another critical aspect of Ethereum development, every operation in your smart contract costs gas, so optimizing your code can significantly reduce transaction costs for your users. Additionally, maintain a robust testing suite that covers all possible scenarios and edge cases. The Ethereum ecosystem is rapidly evolving, so staying connected with the community and keeping up with new developments will help you make informed decisions about your application’s architecture and features.
Next Steps for Learning:
Metaschool offers intensive, hands-on training in a structured web3 learning program. Metaschool’s hands-on Ethereum development courses are offered for free. These self-paced and structured courses guide you to write Smart Contracts in Solidity, build NFTs, and teach you to create your own Ethereum Token in 30 mins with expert instruction and a guided learning environment.
Developers at every stage of their learning journey will benefit from Metaschool’s Ethereum blockchain track and learn to build dApps quickly.
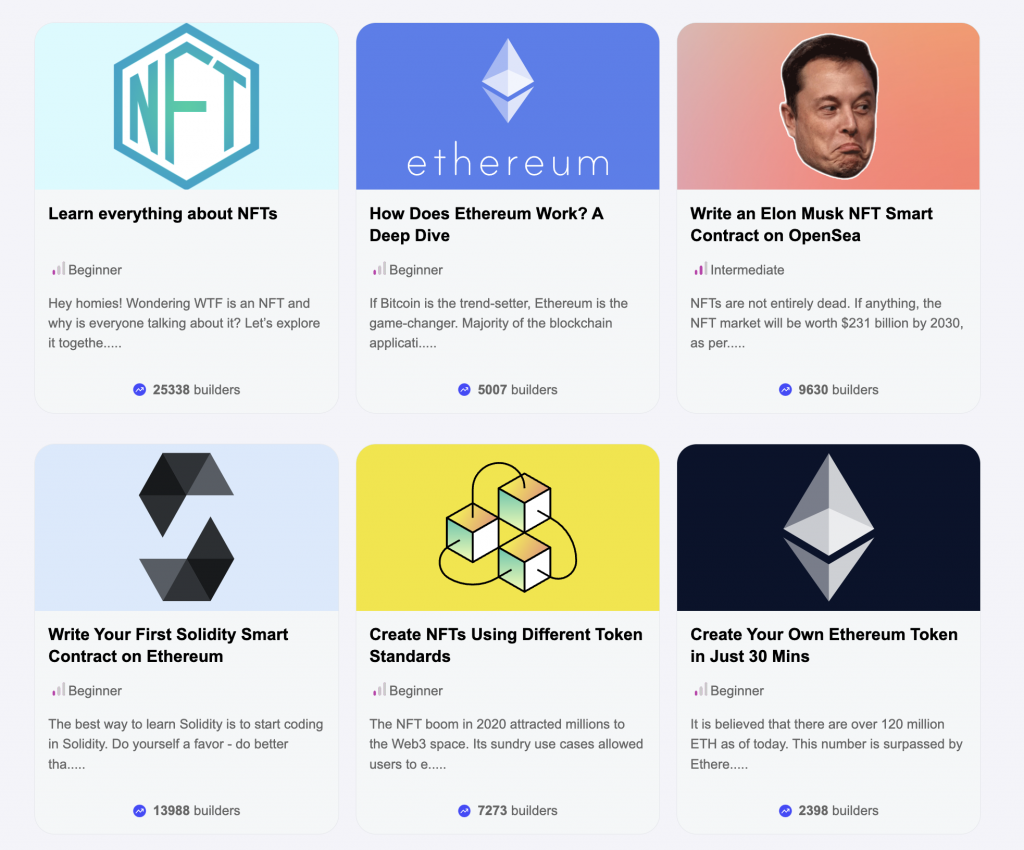
Conclusion
The Web3 ecosystem is constantly evolving, so staying updated with the latest developments and best practices is crucial for building successful dApps. As you continue your journey in Web3 development, several advanced topics are worth exploring to enhance your capabilities as a dApp developer. Diving into advanced smart contract patterns will help you write more sophisticated and secure contracts.
Understanding Layer 2 scaling solutions like Optimistic Rollups and ZK-Rollups is becoming increasingly important as Ethereum scales to meet growing demand. Gas optimization techniques deserve special attention – learning how to write more efficient contracts can save your users significant costs in the long run. Familiarizing yourself with various token standards such as ERC20 for fungible tokens and ERC721 for NFTs will open up new possibilities for your applications. Additionally, exploring decentralized storage solutions like IPFS will help you build truly decentralized applications where both logic and data storage exist outside traditional centralized servers.