Table of Contents
In Solidity, there is no concept of floating point. If you’re reading this then I am assuming that you must know the difference between integers and floating point numbers. A floating point number represents a decimal value like 1.25, while an integer does not. Let’s find out what role floating numbers play in the division.
Division in general
When you divide any two numbers, the result can either be in decimal or integer form. For instance, if you divide 5 by 2, the answer is 2.5, which is a decimal number. On the other hand, if you divide 4 by 2, the answer is 2, which is an integer.
So, how do programming languages account for this difference? Before exploring how Solidity deals with this issue, let’s first examine how Python handles it.
Division in Python
Python is a powerful programming language that provides support for the float data type. This data type allows for the manipulation of floating-point numbers, which can be useful in a variety of computational tasks. In particular, the float data type enables easy division between two floating point numbers, allowing for precise and accurate results in your code.
Let’s look at an example.
a = 5.0
b = 2.0
res = a/b
# output will be 2.5
You can see why having float-type data is beneficial for programming languages. It helps them find and store the right answer of division between any two numbers. This way you find the right answer to division and you don’t lose any data.
Unlike many programming languages, Solidity doesn’t support floating numbers. Let’s find out what answer we get after doing the simple division in Solidity.
🔥 Check this course out: Create Your Own Ethereum Token in Just 30 Mins
The problem of division in Solidity
Solidity offers two types of integers – signed (int
) and unsigned integers (uint
).
Example code #1
Let’s perform a division on unsigned integers in Solidity using the following code.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
contract ExampleContract {
// We will use these variables to perform the division
uint256 num_1;
uint256 num_2;
// constructor function is used to initialize the num_1 and num_2 variables
constructor(uint256 aNum, uint256 bNum) {
num_1 = aNum;
num_2 = bNum;
}
// division function will perform the division functionality
// and return the result
function division() public view returns (uint256) {
return num_1 / num_2;
}
}
This code will give you the following output for the 5 divided by 2 examples.
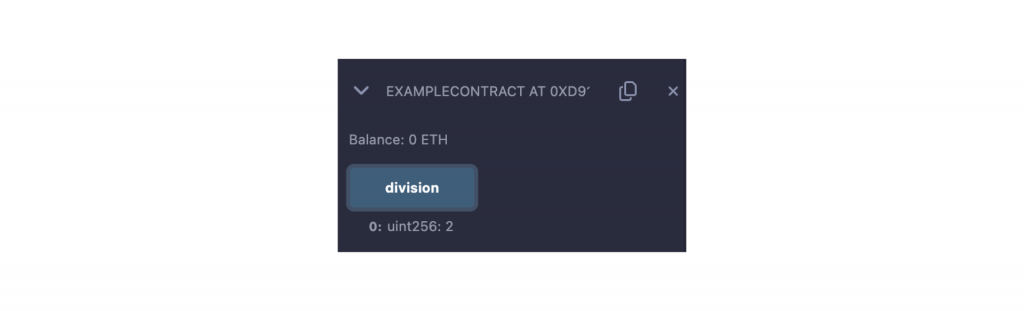
It seems there is an issue here. The division
function provided us with an output of 2 instead of 2.5. This indicates that Solidity rounded up the 2.5 and returned 2 as the result. Moreover, it appears that Solidity does not support floating numbers. You can verify this by testing the code in the Remix IDE.
If you divide 5 by 3, Solidity will round the output to 1 instead of 1.666 on a calculator.
Let’s take another example.
Example code #2
Let’s perform multiplication and division in one line and see what happens.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
contract ExampleContract {
// We will use these variables to perform the division
uint256 num_1;
uint256 num_2;
uint256 num_3;
// constructor function is used to initialize the num_1 and num_2 variables
constructor(uint256 aNum, uint256 bNum, uint256 cNum) {
num_1 = aNum;
num_2 = bNum;
num_3 = cNum;
}
// division function will perform the division functionality
// and return the result
function division() public view returns (uint256) {
return num_1 / num_2;
}
function divAndMul() public view returns (uint256) {
return (num_1 * num_2) / num_3;
}
}
After entering the inputs 2, 3, and 5 for the divAndMul
function in Remix IDE, the following output is obtained:
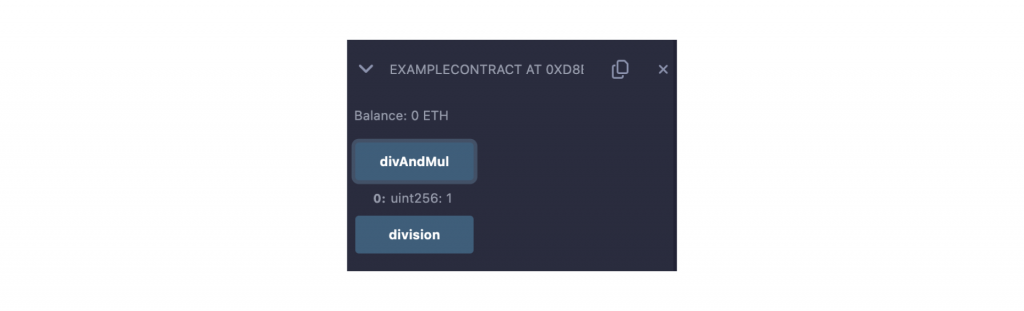
Upon running the Solidity code, it resulted in 1, whereas the calculator gives 1.2 for the same input.
Now, you get the idea of how tricky it is to perform the division in Solidity.
How to prevent division error in Solidity?
The most common way to solve the division error in Solidity is by using a multiplier. A multiplier is an additional variable with a value of 10, 100, or 1000. To solve the issue, we multiply the numerator by the multiplier and then divide the result by the denominator. For example, if we use a multiplier of 100, we would multiply the numerator by 100 and then divide the result by the denominator. Let’s look at the example code for this.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
contract ExampleContract {
// We will use these variables to perform the division
uint256 num_1;
uint256 num_2;
uint256 num_3;
uint256 multiplier;
// constructor function is used to initialize the num_1 and num_2 variables
constructor(uint256 aNum, uint256 bNum, uint256 cNum) {
num_1 = aNum;
num_2 = bNum;
num_3 = cNum;
multiplier = 100;
}
// division function will perform the division functionality
// and return the result
function division() public view returns (uint256) {
return (num_1 * multiplier) / num_2;
}
function divAndMul() public view returns (uint256) {
return (num_1 * num_2 * multiplier) / num_3;
}
}
After entering inputs 2, 3, and 5 respectively, we received the following output in Remix IDE for both functions:
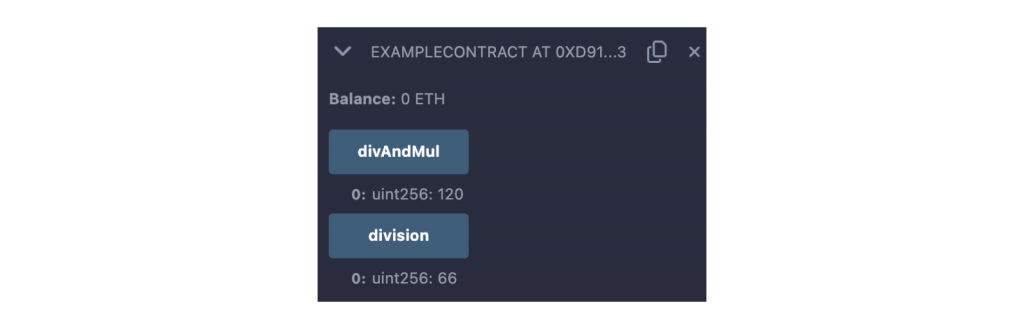
If you divide 120 by 100, you’ll get 1.2, which is what we want. If you divide 66 by 100, you’ll get 0.66, which is also what we want. Therefore, using the multiplier resolved the issue.
🔥 Check this course out: Build a Semi-Fungible ERC404 Tokens’ Marketplace
Conclusion
There are also other methods to resolve this issue, but using the multiplier method is the common and easy way. You can also use libraries like ABDKMath64x64 to resolve the division error.
Try it out, ask us questions, and tell us how it went by tagging Metaschool on Social Media.
Follow us on –
🔮Twitter – https://twitter.com/0xmetaschool
🔗LinkedIn – https://www.linkedin.com/company/0xmetaschool/