Table of Contents
Built-in functions have always been a life-saver for developers, all you need to know is what that particular built-in function is used for, or if you are presented with the use case then which function would do the job. Python has a lot of built-in functions and here we are going to talk about the contains() substring method.
What is a substring?
Before we begin, it is important to know what a substring is.
A substring is a sequence of characters that appears within another string. It is a contiguous segment of the original string, meaning the characters are adjacent and in the same order. For example, in the string "hello"
, "ell"
and "lo"
are substrings.
Does Python have a string contains()
substring method?
The contains()
function in programming is used to check whether a specific substring or pattern is present within a larger string. When you use this function, it returns a boolean value: True
if the substring is found within the string, and False
otherwise. The diagram below demonstrates how the contains()
keyword works.
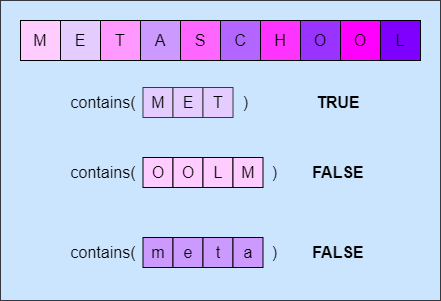
contains()
exampleThe contains()
function has important use cases in text processing tasks, such as searching for keywords, validating input, or filtering data based on content. It helps developers efficiently determine the presence of specific sequences of characters within a string.
Let’s explore the different methods in Python that can be used to check if a string contains a substring.
Checking for a Substring Using the in
Keyword
In Python, you can determine if a string contains a particular substring by using the in
keyword. Let’s understand how to implement in
using a coding example.
text = "Hello, world!"
substring = "world"
if substring <strong>in</strong> text:
print("Substring found!")
else:
print("Substring not found.")
In this example, "world"
is checked to see if it is part of the string "Hello, world!"
. In this case, the in
keyword will return True
.
Additional Methods for String Containment
While the in
keyword is often sufficient, Python provides additional string methods that can be useful for more specific scenarios.
1. str.find(substring)
This method returns the lowest index of the substring if it is found in the string. If the substring is not found, it returns -1
.
index = text.find(substring)
if index != -1:
print(f"Substring found at index {index}.")
else:
print("Substring not found.")
Explanation
Imagine you have a sentence: “The quick brown fox jumps over the lazy dog.” and you want to find the position of the word “fox” within this sentence. If we look through the sentence we find that “fox” starts at the 16th character position. Since the word “fox” is present, we get its starting position, which is 16 (indexing starts at 0). The code then prints “Substring found at index 16.” Instead, if we were searching for the word “cat” the result would be “Substring not found.”
2. str.index(substring)
This method is similar to find()
, but raises a ValueError
if the substring is not found. This can be useful if you want to ensure that the substring is present and handle cases where it is not.
try:
index = text.index(substring)
print(f"Substring found at index {index}.")
except ValueError:
print("Substring not found.")
Explanation
Let’s use the same example “The quick brown fox jumps over the lazy dog.” and we want to find the position of the word “quick”. In this case, this function would behave similar to string.find(substring)
and print “Substring found at index 4.” If we search for a word like “duck” (which isn’t in the sentence), we get an error. In this case, the code prints “Substring not found.”
3. str.startswith(prefix)
and str.endswith(suffix)
These methods check if a string starts with or ends with a given substring, respectively. They are particularly useful for checking prefixes or suffixes.
if text.startswith("Hello"):
print("String starts with 'Hello'.")
if text.endswith("world!"):
print("String ends with 'world!'.")
Explanation
Let’s use the example “Hello, world!” to explain how the code works:
- Checking for Start: The code first checks if the string starts with “Hello”. In our example, “Hello, world!” does indeed start with “Hello”, so it prints “String starts with ‘Hello’.”
- Checking for End: Next, the code checks if the string ends with “world!”. In this example, “Hello, world!” does end with “world!”, so it prints “String ends with ‘world!'”.
If the string did not start with “Hello” or did not end with “world!”, the corresponding messages would not be printed. For instance, if the string were “Goodbye, world!”, the output would be “String ends with ‘world!”.
Bonus Tip
Substring checks are case-sensitive by default. So if you need to perform a case-insensitive check, you can convert both the string and the substring to the same case using str.lower()
or str.upper()
(lower case and upper case respectively) and then check if the string contains the substring.
text = "Hello, World!"
substring = "world"
if substring.lower() in text.lower(): # if "world" in "hello, world"
print("Substring found (case-insensitive).")
else:
print("Substring not found.")
Conclusion
Python’s in
keyword provides a straightforward and effective way to check for substrings within a string in comparison to contains(). For more complex requirements, methods like find()
, index()
, startswith()
, and endswith()
offer additional functionality. Whether you need a simple string containment check or more advanced substring operations, Python’s string-handling capabilities make it easy to work with textual data.
Python is also crucial in the context of blockchain development. Its versatility and powerful libraries make it a good choice for building and interacting with blockchain systems. To understand why Python is so effective for blockchain projects, check out our article, 7 Reasons Why You Should Develop a Blockchain Using Python which explores the numerous advantages of using Python for blockchain development.
FAQs
How can I check if a string contains a substring in Python?
In Python, you can check if a string contains a substring using several methods.
1. Use the in
keyword, which returns True
if the substring is present within the string.
2. Use str.find()
, which returns the index of the first occurrence of the substring or -1
if not found.
3. Use str.index()
, which raises an error if the substring is not found.
4. Use startswith()
and endswith()
to check if a string begins or ends with a specific substring.
Is string containment case-sensitive in Python?
Yes, string containment checks in Python are case-sensitive by default. This means that the check will distinguish between uppercase and lowercase characters. For instance, checking if "hello"
is in "Hello World"
will return False
because of the case difference. To perform a case-insensitive check, you should convert both the main string and the substring to the same case using str.lower()
or str.upper()
.
Can I check for multiple substrings in a single operation?
Python does not provide a built-in method to check for multiple substrings in a single operation. However, you can achieve this by iterating through a list of substrings and checking each one individually. For example, you can use a loop with the in
keyword to check if any of the substrings are present in the main string.