Table of Contents
In Python, strings are a fundamental data type used to represent and manipulate text. Understanding how to work with the length of strings is crucial for many programming tasks, from validating input to processing text data. In this guide, we will explore how to determine and utilize the string lengths effectively.
Getting the Length of a String
To find out string length in Python, you can use the len()
function. This built-in function returns the number of characters in the string, including letters, numbers, spaces, and punctuation.
Let’s see how we can implement this function:
# Get the length of the string
length = len(text)
# Print the length
print("The length of the string is:", length)
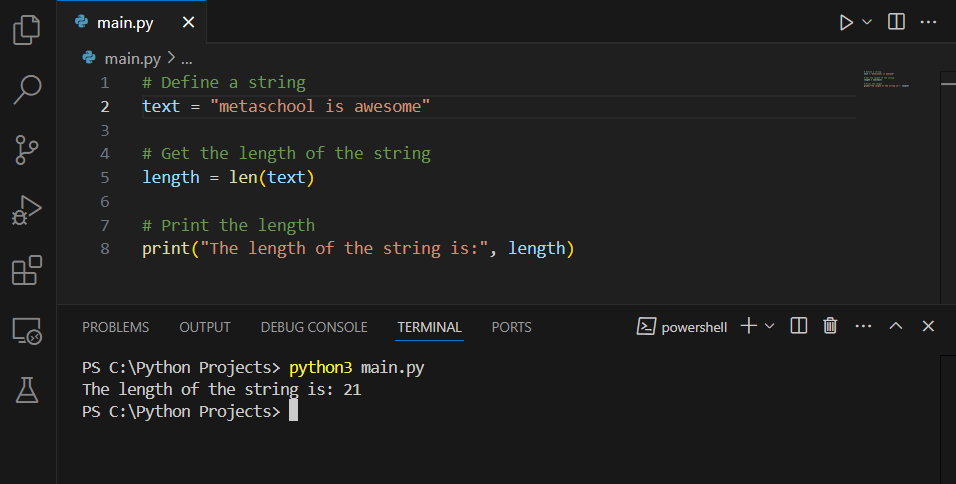
Applications of String Length
1. User Input Validation
Ensuring that user input meets certain length requirements is crucial for data integrity and security. For example, validating passwords to ensure they are of a minimum length helps enforce security policies. To enhance security, a system might require passwords to be of a minimum length. Additionally, it might provide feedback if the password length is insufficient.
def validate_password(password):
min_length = 8
if len(password) < min_length:
return f"Password must be at least {min_length} characters long."
return "Password is valid."
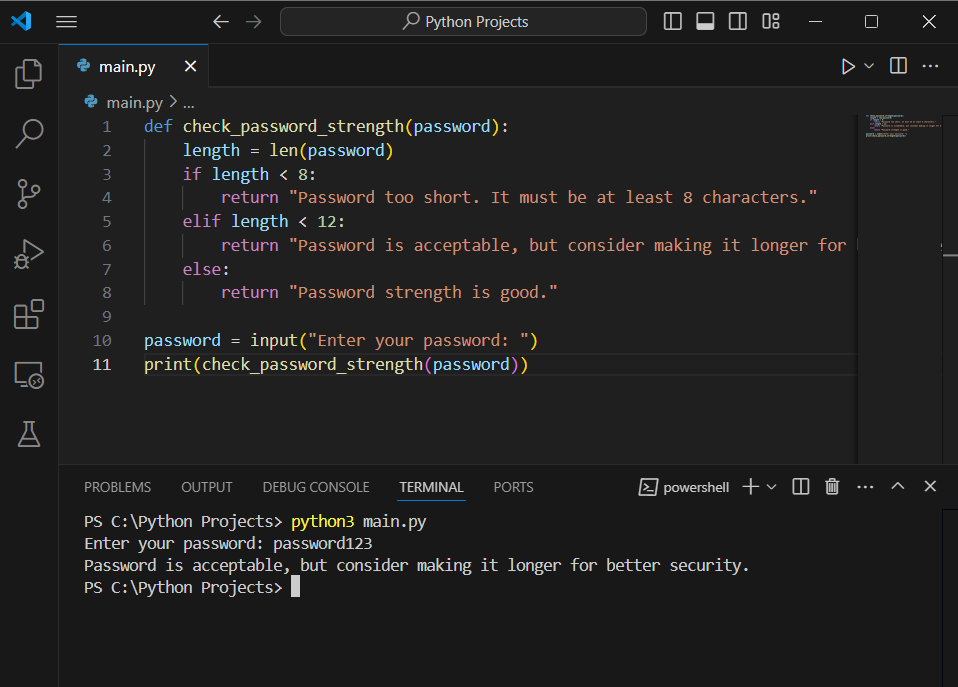
3. Find Longest/Shortest String
When working with lists of strings, you might want to find the longest or shortest string. Knowing the length of each string helps achieve this.
def find_longest_shortest(strings):
longest = max(strings, key=len)
shortest = min(strings, key=len)
return longest, shortest
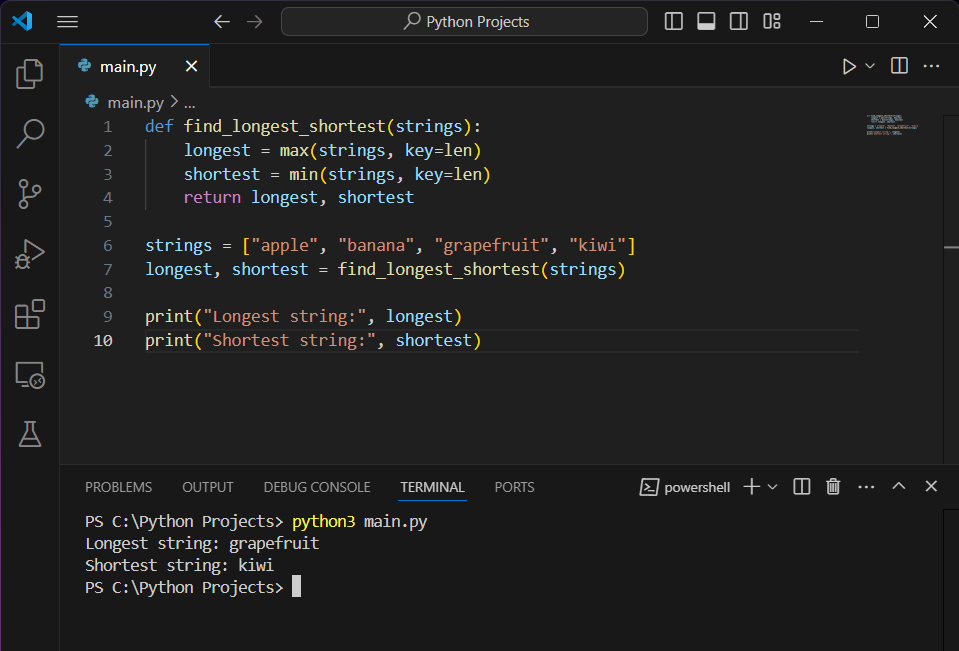
4. Creating Pagination
When dealing with large amounts of text or data, pagination is often used to divide content into manageable sections. Knowing the length of the text helps determine how to split it across pages.
def paginate_text(text, page_size):
pages = [text[i:i+page_size] for i in range(0, len(text), page_size)]
return pages
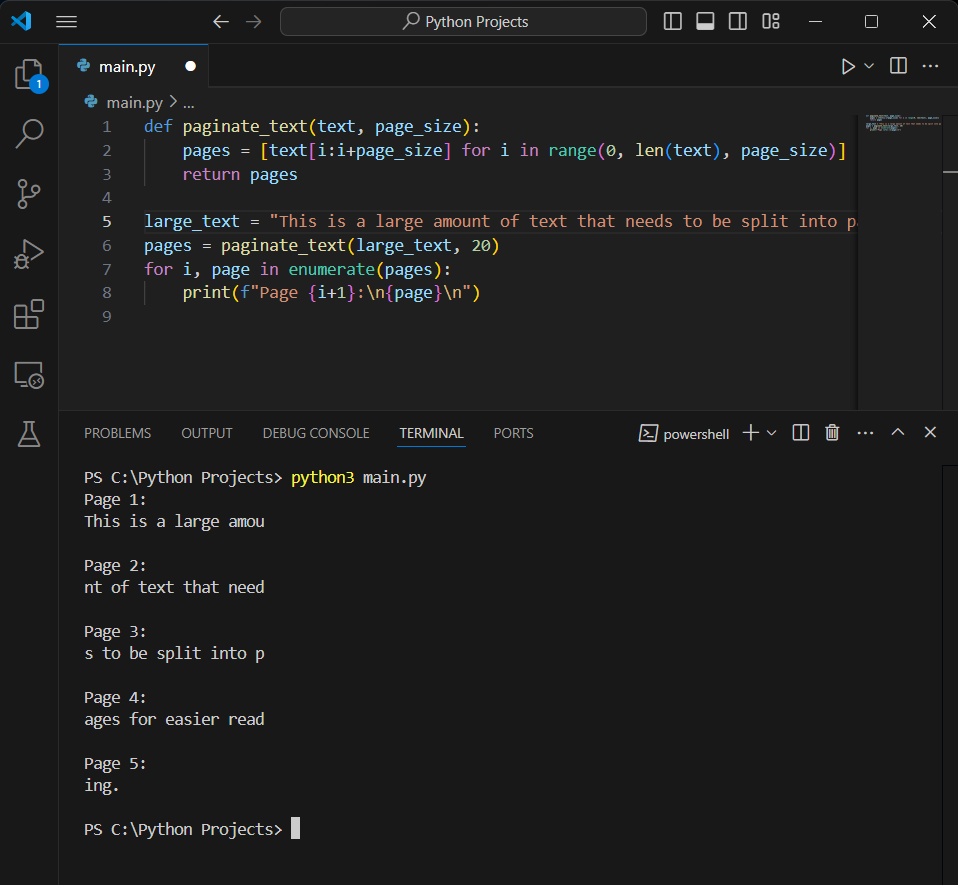
Special Considerations
For strings containing multibyte characters (like emojis or characters from non-Latin scripts), len()
still counts the number of characters, but the byte length might differ:
text = "😊"
print("Character length:", len(text)) # Outputs: 1
Strings with Unicode characters can have different lengths based on normalization forms. For instance, the Unicode representation of some characters might differ:
text1 = "é" # e followed by an accent mark (combined)
text2 = "é" # e with an accent mark (single character)
print(len(text1)) # Outputs: 2
print(len(text2)) # Outputs: 1
To handle such cases, consider normalizing strings before processing:
import unicodedata
text = "é" # Example with combining characters
normalized_text = unicodedata.normalize('NFC', text)
print(len(normalized_text)) # Outputs: 1
Conclusion
The len()
function in Python provides a straightforward way to determine the length of a string. Whether you’re validating user input, manipulating text, or analyzing data, understanding string length is a foundational skill in programming. By mastering this concept, you’ll be better equipped to handle various text processing tasks and ensure your programs operate as expected.
In addition to these fundamental programming skills, Python’s versatility extends into more specialized areas, such as blockchain development. Its powerful libraries and adaptability make Python an excellent choice for building and interacting with blockchain systems. To explore why Python is so effective for blockchain projects, check out our article, 7 Reasons Why You Should Develop a Blockchain Using Python, which delves into the many advantages of using Python for blockchain development.
FAQs
How does Python handle the length of Unicode strings?
Python’s len()
function accurately counts the number of Unicode characters, even if they are represented by multiple bytes. For example, len("😊")
returns 1
, as it counts the emoji as a single character.
What should I do if my string slicing isn’t working as expected?
Ensure your slice indices are within the string’s length. Python handles out-of-bound indices gracefully. For example, text[0:20]
on a short string will return the entire string without error.
How can I find the longest and shortest strings in a list?
Use max()
and min()
with the key=len
argument. For example:longest = max(strings, key=len)
shortest = min(strings, key=len)
This efficiently identifies the longest and shortest strings based on length.