Table of Contents
In Solidity, “msg.sender” is something that is used frequently and it is important to get familiar with it, know what exactly it does, and what is it used for.
Introduction to msg.sender
So, “msg.sender” is a dynamic global variable in Solidity that you can access anywhere in the code without importing anything. This variable is filled automatically when any transaction happens. It is called a dynamic variable because it can change throughout the transaction process.
“msg.sender” represents the address of the account that called the function present within the smart contract. What if one smart contract calls another smart contract? In this case, the “msg.sender” will have the address of the calling smart contract instead of an account address.
For example, if contract A calls contract B, then msg.sender
inside of contract B will have the address of contract A. And if a certain wallet calls the contract then the msg.sender
will have the wallet address.
msg.sender
is used wherever we need to track the source of the transaction within the smart contract. So, “msg.sender” can be the address of a smart contract or the address of the account.
🔥 Check this out: Build in Public
Solidity msg.sender example
Here is how you can use msg.sender
in your Solidity code.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
contract ExampleContract {
address private _owner;
constructor() public {
_owner = msg.sender;
}
function getOwner() public view returns (address) {
return _owner;
}
}
Explanation
Let’s look at what we did here.
- First of all, we defined the version for our Solidity compiler.
- Then, we defined the contract named
ExampleContract
. - Inside of the contract, we defined a private variable
_owner
of theaddress
type. - Inside the
constructor
function, we initialized the_owner
variable with themsg.sender
address. Now, whoever will call the contract or define the contract will be its owner. - To get the
_owner
address, we define thegetOwner()
function inside of theExampleContract
that returns the value of_owner
variable.
🔥 Check this course out: Write an Elon Musk NFT Smart Contract on OpenSea
Output
After deploying the contract on Remix IDE, you’ll see a button getOwner
that will allow you to view the msg.sender
value. Let’s look at where you can find the getOwner
button.
Here’s what the output looks like in my case:
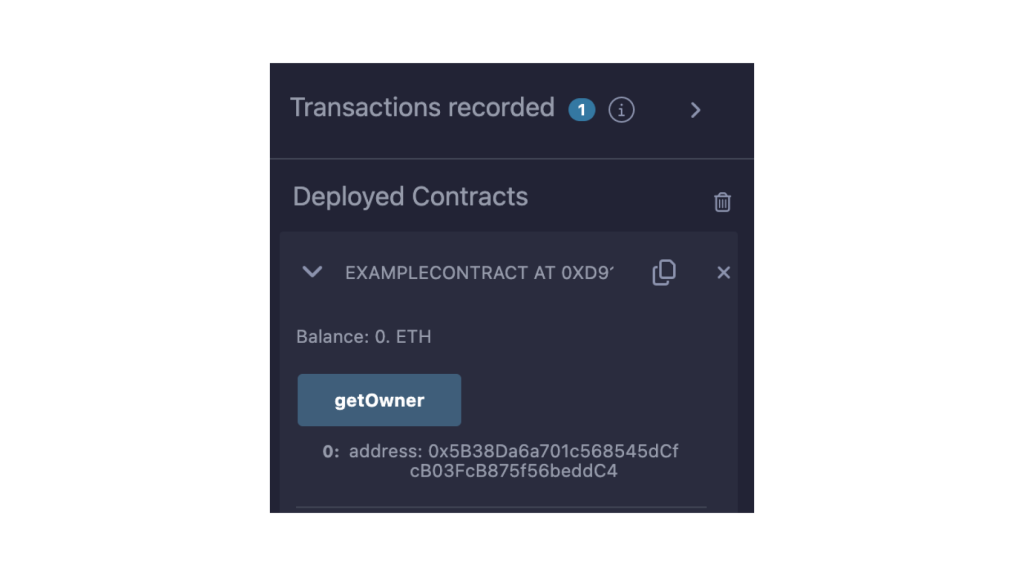
🔥 Check this course out: Create Your Own Ethereum Token in Just 30 Mins
Conclusion
Note that, “msg.sender” can save either the contract or the wallet address. This means that if one contract calls for another contract, the 2nd contract will receive the 1st contract address as msg.sender
. This helps track the source of the transaction within the smart contract.
Tried this out? Let us know how it went by tagging Metaschool on your Social Media!
Follow us on –
🔮Twitter – https://twitter.com/0xmetaschool
🔗LinkedIn – https://www.linkedin.com/company/0xmetaschool/