Table of Contents
Let’s explore the easiest way to convert the Solidity uint to string.
Convert uint to string using OpenZeppelin library
The easiest way to convert the uint to a string is using String.sol
contract from the OpenZeppelin library. String.sol
offers a function called toString
that can easily convert any uint to a string. Here’s how toString
works.
Strings.toString(myUint)
🔥 Check out our latest building program: Build in Public
uint to string example
Let’s look at the complete example now.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
// importing String.sol from openzeppelin library
import "@openzeppelin/contracts/utils/Strings.sol";
contract ExampleContract {
function convertToString(uint num) public pure returns (string memory)
{
string memory strNum; // define string type memory variable
strNum = Strings.toString(num); // converts unsigned int to a string
return strNum; // return string
}
}
Explanation
- First of all, we defined the version for our Solidity compiler.
- We imported the
Strings.sol
contract from the OpenZeppelin library. - Created a contract named
ExampleContract
. - Inside the contract, we defined a public function named
convertToString
that takesuint
typenum
as an input and returns a string. - The function first defines the string type memory variable
strNum
. - Next, we call the
Strings.toString(num)
function and stored the value it returns to thestrNum
. - At last, we return the
strNum
.
Running our example in Remix IDE
Let’s run our example in Remix IDE and see what it returns.
Output
Here’s what the output looks like in my case.
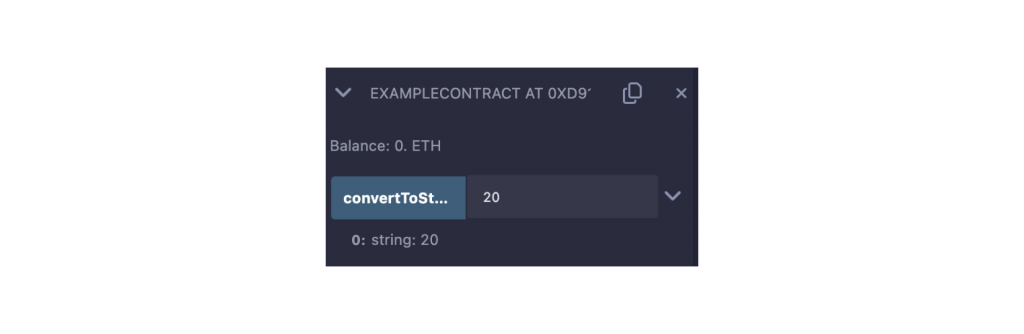
🔥 Check this course out: Write an Elon Musk NFT Smart Contract on OpenSea
Conclusion
Importing the OpenZeppelin String.sol
contract in your solidity code can easily help you convert the uint type variable to the string.
Try it out and let us know how it went by tagging Metaschool on Social Media.
Follow us on –
🔮Twitter – https://twitter.com/0xmetaschool
🔗LinkedIn – https://www.linkedin.com/company/0xmetaschool/