Table of Contents
Artificial Intelligence is changing how we think, operate, and act with machines. Here we will build an AI-powered personal fitness coach who can tailor a personalized workout plan based on your lifestyle, future goals, and current fitness level. It is possible in this era of Artificial Intelligence without leaving your home. An AI-powered personal fitness coach can generate personalized workout plans using GPT-4, a remarkable language model from OpenAI.

In this article, we’ll build an AI-powered personal fitness coach who can be a fun workout buddy throughout your fitness journey. The making of an AI-powered personal fitness coach can be a game changer in your life. It can enable a vast majority of users to start their journey having no clue about where to start from. While some people take help from Google for their fitness plan others may follow trending YouTube channels. But usually, these sources offer one generic plan for everyone. This is where the problem starts because every human has a unique body, different goals, and strengths or weaknesses as compared to others.
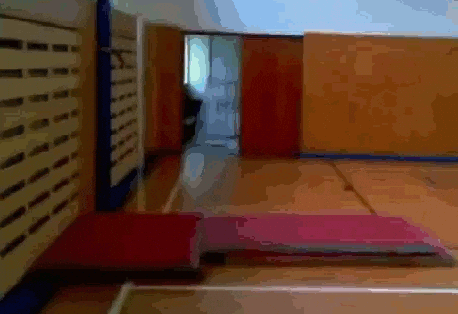
To solve this problem, we need personalized assistance. This is where AI comes to the rescue to support you in your fitness journey! An AI-powered personal fitness coach can recommend a workout plan based on your input like age, weight, and fitness level. Seems cool, right? Let’s get into it and start building it from scratch.
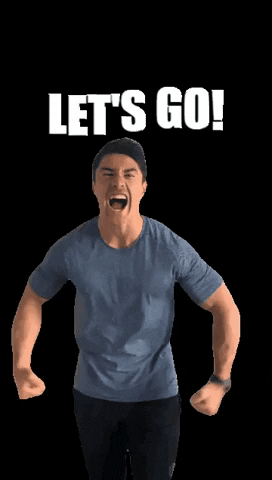
Let’s walk through each step to make it easy for you to follow.
Setting up the environment
Alright, buddy, the first thing we need to do is to set up a development environment. We need to set up an environment that is suitable for AI integration and web application development. We’re going to use Flask for our web framework, trust me it’s super easy to use, and Python as a background programming language. We’re going to use Flask to create a simple web app that will interact with GPT-4 API. Have you ever heard of Flask earlier? If don’t, no worries.
Flask is a web framework that supports us when we just want to get things done. It is a lightweight web framework to create the web interface. We’ll be using the Flask server to run our application on top of our local server. To ensure we can use Python and Flask seamlessly, let’s first install these on our system.
Install Python and Flask
Firstly we need to ensure Python is installed on our system. Use the terminal to check if it is already installed by running the following command on our terminal.
python --version
If you see a version number just pops up, congrats, you’re good to go. If it’s not installed, don’t panic, head over to python.org and download it on your system.
Once it is sorted, now open your terminal and create a new project directory as follows:
mkdir fitness-coach
cd fitness-coach
Now let’s install Flask and OpenAI, the backbone of our system as follows.
pip install flask openai
Initialize the OpenAI API
Now let’s bring the brain of our system—The OpenAI API. To use OpenAI’s API, we’ll need to sign up over the OpenAl platform and create an account. After signing in, move towards the API keys section and generate a new key. Keep this key safe because it’s your golden ticket to access the GPT models.
If you face any difficulty during the signup and API key generation follow this guide to use OpenAI API to learn more about OpenAI API’s. We also need to ensure our API is not exposed throughout the creation process.
Securing our OpenAI API
Once you get an OpenAI’s AP, now it’s time to ensure the API key remains secured throughout the process. To securely use our API, we can set it as an environment variable. We need to create a .env
file in our project directory by using the following command:
# For Mac users
echo "OPENAI_API_KEY=your_api_key_here" > .env
# For Windows users
echo OPENAI_API_KEY=your_api_key_here > .env. # Using Command Prompt
echo "OPENAI_API_KEY=your_api_key_here" > .env. # Using PowerShell
In these commands replace your_api_key_here
with your actual API key and execute the respective command based on your system preference.
To ensure our application can read this API key. We can use python-dotenv
package to manage this process as follows:
pip install python-dotenv
In this way, we can keep it secret, just like you don’t tell anyone about your cheat meals. 🍕😜
Creating our backend
In our project folder, create a new Python file called app.py
. We can create it using the following command:
# For Mac user
touch app.py
# For Windows user
echo. > app.py # Using command prompt
New-Item -Path app.py -ItemType File # Using PowerShell
This file will serve as the brain of our operations. Open app.py
and add the following code:
from flask import Flask, render_template, request
import openai
from dotenv import load_dotenv
import os
# Load environment variables from .env file
load_dotenv()
openai.api_key = os.getenv("OPENAI_API_KEY")
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
# Route to handle form submission and generate the workout plan
@app.route('/generate_plan', methods=['POST'])
def generate_plan():
# Collecting user input from the form
user_data = {
"age": request.form.get('age', ''),
"weight": request.form.get('weight', ''),
"goal": request.form.get('goal', ''),
"fitness_level": request.form.get('fitness_level', ''),
"workout_frequency": request.form.get('workout_frequency', '')
}
# Generating the workout plan using GPT-4
try:
plan = generate_workout_plan(user_data)
except Exception as e:
# Handle any errors that occur during the OpenAI API call
plan = f"Error generating workout plan: {str(e)}"
# Rendering the result in the same HTML page
return render_template('index.html', plan=plan)
def generate_workout_plan(user_data):
# Creating a prompt for GPT-4 based on user input
messages = [
{"role": "system", "content": "You are an AI-driven fitness coach."},
{"role": "user", "content": f"Create a personalized weekly workout plan for the following individual:\\n\\n"
f"Age: {user_data['age']}\\n"
f"Weight: {user_data['weight']} kg\\n"
f"Goal: {user_data['goal']} (e.g., weight loss, muscle gain, endurance)\\n"
f"Fitness level: {user_data['fitness_level']} (e.g., beginner, intermediate, advanced)\\n"
f"Workout frequency: {user_data['workout_frequency']} times per week.\\n\\n"
"Provide day-wise workout plan with warm-ups, exercises, sets, reps, and advice for motivation and recovery."}
]
# Using OpenAI's GPT-4 chat model to generate the workout plan
response = openai.ChatCompletion.create(
model="gpt-4", # Specify the chat model
messages=messages,
max_tokens=300, # Adjust the token size based on the complexity of the response
temperature=0.7 # Creative freedom for GPT-4
)
# Extracting the workout plan from GPT-4's response
workout_plan = response['choices'][0]['message']['content'].strip()
return workout_plan
if __name__ == "__main__":
app.run(debug=True)
Let’s pause and understand what’s happening inside app.py
file. We started by importing some packages like Flask
for our web framework and openai
for interacting with the GPT-4 model. We load our API key from the environment variables using dotenv
. Following setting up our web routes, we initialized two routes "/"
for displaying the homepage and "/generate_plan"
to process the information gathered from the form, passing it to GPT-4, and displaying the workout plan.
Once our backend is completed, let’s talk about the form that will gather information from the user.
Setting up the user interface
Fitness apps usually have a sleek and simple interface as no one likes a complicated form. Let’s try to keep it clean, just like our well-balanced diet. 💪
HTML structure
Inside our project folder, create a new folder named templates
. Inside this new folder, create an HTML file named index.html
.
mkdir templates
touch templates/index.html
Following is the HTML code used to set up our form. Here it allows users to input their information.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AI Fitness Coach</title>
<link rel="stylesheet" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<div class="container">
<h1>AI-Driven Fitness Coach</h1>
<form action="/generate_plan" method="POST">
<label for="age">Age:</label>
<input type="number" id="age" name="age" required>
<label for="weight">Weight (in kg):</label>
<input type="number" id="weight" name="weight" required>
<label for="goal">Fitness Goal:</label>
<select id="goal" name="goal" required>
<option value="Weight Loss">Weight Loss</option>
<option value="Muscle Gain">Muscle Gain</option>
<option value="Endurance">Endurance</option>
</select>
<label for="fitness_level">Fitness Level:</label>
<select id="fitness_level" name="fitness_level" required>
<option value="Beginner">Beginner</option>
<option value="Intermediate">Intermediate</option>
<option value="Advanced">Advanced</option>
</select>
<label for="workout_frequency">Workout Frequency (times per week):</label>
<input type="number" id="workout_frequency" name="workout_frequency" required>
<button type="submit">Get Personalized Workout Plan</button>
</form>
<!-- Display the generated workout plan -->
{% if plan %}
<div class="plan-result">
<h2>Your Personalized Workout Plan</h2>
<pre>{{ plan }}</pre>
</div>
{% endif %}
</div>
</body>
</html>
The HTML structure provides dropdown-based 5 input fields, which will allow users to enter their data into our AI-powered personal fitness coach just like the following.

You know how important appearance is for any app, right? We need to ensure our AI-powered personal fitness coach provides good aesthetics that motivate you to keep going. In the next section, we’re going to add some life-using colors to our AI-powered personal fitness coach.
CSS based styling
Let’s style our boring webpage by playing with some colors around it. First, let’s create a folder in the project directory named as static
, and inside it create a file called style.css
using the following:
mkdir static
# For MAC users
touch static/style.css
# For windows users
cd static
echo. > style.css # Using command prompt
New-Item -Path style.css -ItemType File # Using PowerShell
Once styles.css
file is created paste the following code inside it:
body {
font-family: Arial, sans-serif;
background-color: #f5f5f5;
margin: 0;
padding: 0;
}
.container {
width: 60%;
margin: 50px auto;
background-color: white;
padding: 20px;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.1);
border-radius: 8px;
}
h1 {
text-align: center;
color: #333;
}
form {
display: flex;
flex-direction: column;
gap: 15px;
}
label {
font-weight: bold;
}
input, select, button {
padding: 10px;
font-size: 16px;
border: 1px solid #ddd;
border-radius: 5px;
}
button {
background-color: #28a745;
color: white;
border: none;
cursor: pointer;
}
button:hover {
background-color: #218838;
}
.plan-result {
margin-top: 20px;
padding: 15px;
background-color: #e9ecef;
border-left: 5px solid #28a745;
}
This code provides a new modern and clean look. We have used containers to center align the overall design and use some padding to ensure our interface is user-friendly.
Now that we’ve set up the front end and back end of the AI-powered personal fitness coach, it’s time to test it out by running our applications in the upcoming section.
Running our application
Now it’s time to run our application and utilize our AI-powered personal fitness coach in action.
Start our Flask development server by running the following command:
python app.py
It will start the server and launch the app at http://127.0.0.1:5000/
. You’ll see the interface of our AI-powered personal fitness coach. Enter all the inputs, click on “Get Personalized Workout Plan” and a personalized workout plan is provided in no time, thanks to the power of OpenAI!
Congratulations! You’ve built a simple but powerful AI-powered personal fitness coach. It is tailored to your specific goals and needs. The powerful GPT-4 model allows you to create a highly customized plan without the need to hire a personal trainer or go through tons of online resources to design one for yourself.
Whether you’re a seasoned fitness freak or someone just getting started on your fitness journey, this AI-powered personal fitness coach is your workout buddy, ready to help you crush your goals.🏋️♀️
So, what are you waiting for, Ace through your AI fitness coach and start making the gains today. If you want to take this project to the next height, here are a few suggestions:
- Track the progress of the users, show them their progress over time, and use animations to keep them motivated.
- Convert this web app to a mobile app using frameworks like Flutter or React Native.
- Integrate videos with the app, along with the generated exercises provide them with the videos as well.
Explore more on Metaschool
If you found building an AI-powered personal fitness coach helpful, you might be interested in exploring more hands-on projects and courses to expand your skills. Check out our other resources on Metaschool:
- Build a YeBot with OpenAI API: Learn how to create a sophisticated chatbot using OpenAI’s powerful API, perfect for enhancing your understanding of AI-driven applications.
- How to Build a Sentiment Analysis Tool Using AI: Dive into building a sentiment analysis tool is a perfect solution to the never-ending task of manually sorting hundreds of comments and trying the understand the sentiment of your users.
Explore these courses and more to continue your journey in AI on the Metaschool platform!
FAQs
What kind of fitness goals can the AI-powered personal fitness coach support?
The AI-powered personal fitness can provide tailored plans for various goals like muscle gain, weight loss, or endurance improvement as per your specific needs.
Can I update my workout plan if my fitness goals or frequency changes with time?
Absolutely! We can cover such cases. you can input new data such as updated fitness level, frequency, or goals. Our AI-powered personal fitness coach can generate fresh and personalized plans accordingly.
How many workouts per week does the AI-powered personal fitness coach recommend?
An AI-powered personal fitness coach can generate plans based on your preferred workout frequency, You can specify it when submitting your data.