Table of Contents
Solidity was designed as the programming language for writing smart contracts on the Ethereum blockchain. It offers developers a range of tools to interact with the blockchain. Among these is a global variable called block.timestamp
that provides the current timestamp of the block being mined.
What is block.timestamp?
block.timestamp
tells you the exact moment since the Unix epoch (more on this later) when a specific block was created on the blockchain. This is often used in smart contracts to handle time-sensitive logic, such as checking if a deadline has passed or tracking when an event occurred. However, it is important to understand that block.timestamp is not perfectly precise and can be manipulated by miners within a small range, so it should be used cautiously, especially for time sensitive applications. Despite its limitations, it’s a useful tool for adding basic timing functionality to your contracts.
What is the Unix epoch?
The Unix epoch is a standard point in time used as a reference for computing and measuring time in many programming languages and systems. It is defined as January 1, 1970, at 00:00:00 UTC (Coordinated Universal Time).
In this system, time is represented as the number of seconds that have elapsed since the Unix epoch. For example, a timestamp of 0 corresponds to the exact moment of the Unix epoch (January 1, 1970, 00:00:00 UTC). The Unix epoch is widely used because it provides a simple, consistent way to represent time across different platforms and programming environments. It is especially useful for time calculations and comparisons in systems like databases, operating systems, and blockchain platforms.
Example Contract Using block.timestamp
pragma solidity ^0.8.0;
contract TimestampExample {
uint256 public deploymentTimestamp;
constructor() {
deploymentTimestamp = block.timestamp;
}
function hasTimePassed(uint256 secondsPassed) public view returns (bool) {
uint256 currentTimestamp = block.timestamp;
return currentTimestamp >= deploymentTimestamp + secondsPassed;
}
}
Feel free to make changes to this code and experiment with different use cases for
block.timestamp
. You can compile and deploy this smart contract on Remix IDE. If you want help with how to run code on this online IDE, checkout this comprehensive tutorial.
Code Explanation
The code above defines a smart contract called TimestampExample
.
pragma solidity ^0.8.0
is a directive to ensure that the code is compatible with the specified Solidity versions (0.8.0 and above).deploymentTimestamp
is a public variable that stores the timestamp of the block when the contract is deployed. It is initialized in the constructor usingblock.timestamp
.hasTimePassed
is a function that accepts a single input,secondsPassed
which represents the time elapsed in seconds (Solidity also supports other time units like minutes andhours
). This function compares the current block’s timestamp with the sum ofdeploymentTimestamp
andsecondsPassed
to determine if the specified duration has elapsed since deployment. If the condition is met, the function returnstrue
; otherwise, it returnsfalse
.
This simple contract can be used as a base structure for implementing smart contracts with time-based logic, such as unlocking new features or triggering actions after a delay.
Considerations and Security Implications for block.timestamp
The use of block.timestamp
in Solidity offers convenience for handling time-sensitive operations, but it comes with several considerations and security implications that developers need to account for.
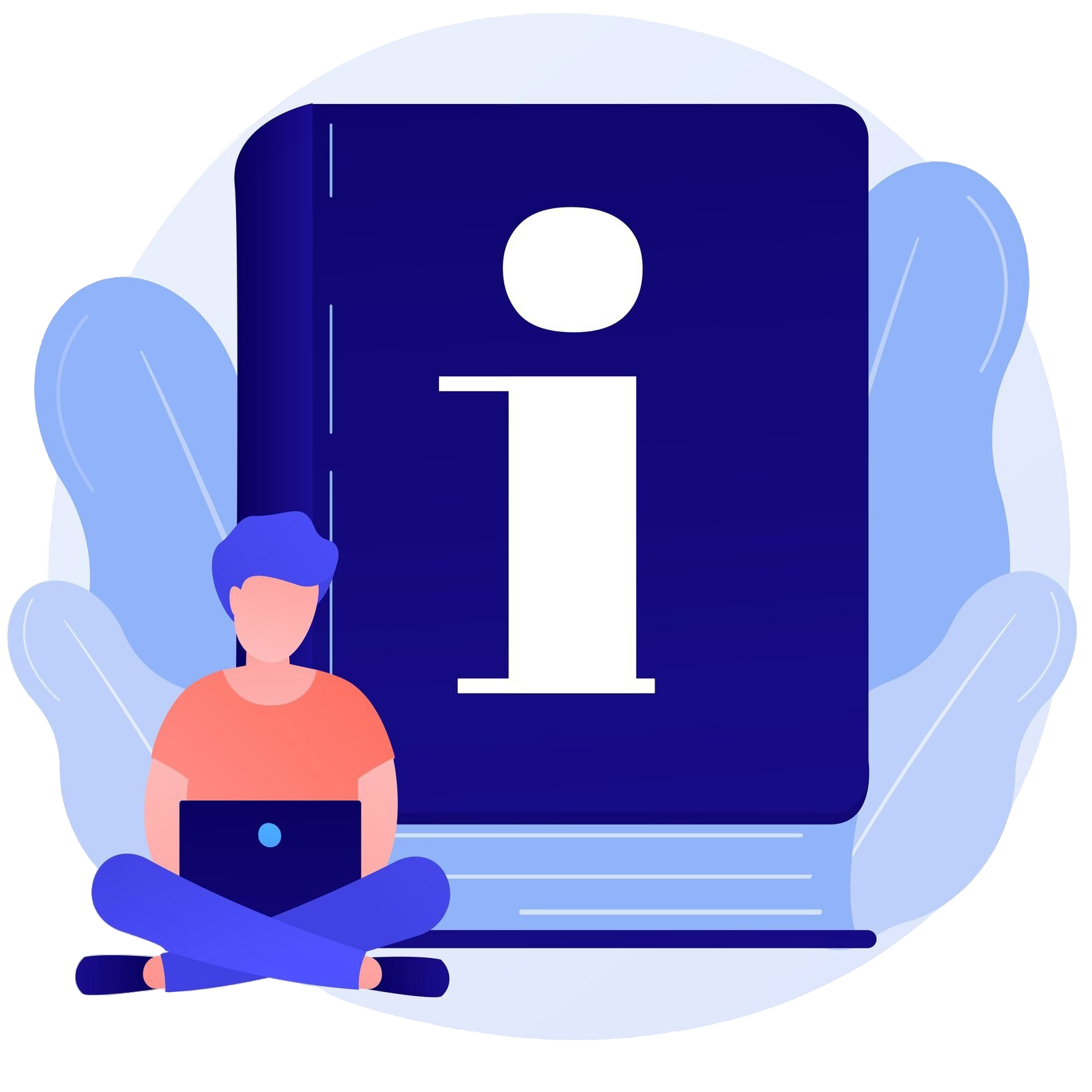
- Miner Manipulation:
Miners have the ability to slightly adjust theblock.timestamp
within a reasonable range, usually a few seconds. This is because block timestamps are not strictly enforced to be precise but must follow the protocol’s rules, such as being greater than the previous block’s timestamp. This manipulation can potentially be exploited in certain applications, such as games or financial contracts, where precise timing is crucial. - Variance:
block.timestamp
is inherently imprecise and subject to minor variances due to the distributed nature of blockchain networks. Relying on it for highly accurate time-dependent operations, such as scheduling or expiry checks, can lead to unintended behavior, especially in edge cases where small discrepancies might have significant consequences. - Best Practices for Enhanced Security:
To mitigate risks associated withblock.timestamp
, developers should follow these best practices:- Avoid Critical Dependencies: Do not rely solely on
block.timestamp
for critical time-sensitive decisions like randomness generation, as this can be exploited by adversaries. - Introduce Buffers: When designing time-dependent logic, introduce reasonable buffers or tolerances to account for potential variances and manipulations.
- External Time Oracles: For applications requiring highly accurate and tamper-proof time data, consider integrating external time oracles. These services provide verified and consistent timestamps, ensuring greater reliability and security in time-sensitive contracts.
- Avoid Critical Dependencies: Do not rely solely on
By understanding the limitations and risks of block.timestamp
, developers can design contracts that minimize vulnerabilities while leveraging its utility for non-critical timing operations.
Conclusion
In conclusion, block.timestamp
is a valuable tool in Solidity for introducing time-related functionality in smart contracts. Developers can leverage this global variable to build dynamic and versatile decentralized applications on the Ethereum blockchain. However, it is essential to be mindful of its limitations and consider additional security measures for critical use cases.
Want to join the Solidity Mastery Fellowship at Metaschool?
Join the waitlist for a 6-week accelerated learning path for developers to become highly efficient in Solidity. This fellowship is the place to be for ambitious builders and developers.
Related Reading:
- Fastest Way To Learn Solidity For Free – 2024 Guide
- The Ultimate Guide to Solidity – 2024
- What is a Solidity interface? Explained with examples
- Understand mappings in Solidity
FAQs
What is block.timestamp
in Solidity, and how is it used?
block.timestamp
is a global variable in Solidity that returns the Unix timestamp of the current block, measured in seconds since January 1, 1970. Developers use it to implement time-dependent logic in smart contracts, such as setting time-based conditions, scheduling events, or enforcing deadlines. For example, you might use block.timestamp
to ensure a function can only be executed after a certain time has passed since contract deployment.
Can block.timestamp
be manipulated, and what are the security implications?
Yes, block.timestamp
can be slightly manipulated by miners or validators. They have the discretion to adjust the timestamp within a reasonable range to some extent. This potential manipulation poses security risks, especially for applications requiring precise timing or randomness. For instance, using block.timestamp
for random number generation can be exploited by malicious actors. Therefore, it’s advisable to avoid relying on block.timestamp
for critical time-sensitive operations and consider using more reliable sources like external time oracles when precision is essential.
Is block.timestamp
measured in seconds or milliseconds, and how does it affect time calculations in smart contracts?
block.timestamp
is measured in seconds since the Unix epoch (January 1, 1970). This means that when performing time calculations in smart contracts, you should account for time intervals in seconds. For example, to set a condition for a 24-hour period, you would use 24 * 60 * 60
seconds. Understanding this is crucial for implementing accurate time-based logic in your contracts.