Table of Contents
The Node.js crypto module is a built-in module that provides a variety of cryptographic functions for Node.js applications, which are written in JavaScript. JavaScript is a programming language that is primarily used for creating web applications, and Node.js is a JavaScript runtime that allows developers to run JavaScript code on a server.
What is the Node.js Crypto Module?
The crypto model is part of the core libraries of Nodejs and doesn’t require any external dependencies. It provides developers with functions to perform cryptographic operations. The cryptographic operations supported on Nodejs crypto module are:
- Hashing data
- Encryption and decryption using both symmetric and asymmetric encryption algorithms
- Generating cryptography signatures and verifying
- Creating random numbers and keys
These features make nodejs crypto module an important library for developers working with cryptography and blockchain applications and use cases.
How does it work?
The Node.js crypto module provides a collection of cryptographic functionality like creating hashes, signing and verifying messages, and encrypting and decrypting data. These functions can be used to perform different types of encryption, decryption, signing, and hashing operations.
It is implemented in C++ and it’s considered to be relatively fast and efficient. The crypto module is a great tool for adding security to your application by performing cryptographic operations on sensitive data.
Features of Nodejs Crypto Module
- Hashing and HMAC: Input arbitrary size data and get fixed-sized digests. It has usage for data integrity and verification.
- Symmetric Encryption: You can encrypt data using a key and decrypt it with the same key to reveal original data.
- Asymmetric Encryption: In this form of encryption, there’s a key pair of public and private keys. Private keys are used to decrypt while it’s public key pair used to encrypt data.
- Digital signatures: Generate and verify digital signatures for data to ensure integrity and authenticity.
- Random Number Generation: Generate secure random numbers and keys (random numbers are used as keys and kept secret).
- Password-Based key Derivation: Safely derive keys from password.
Plain Text: For example, the passwords that we type are plain text. Anything that we type or write and is humanly readable is plain text. It contains characters, numbers, and special characters.
Cipher Text: For example, “oiewhrgfsd23049854remf^&dlj”, this random strong of text, numbers, and special characters is non-readable and not human understandable. It is generated randomly or from a text input.
Uses of Node.js crypto Module and Additional Crypto NPM Package
1. The crypto
module is a built-in module in Node.js, which means it is included with the Node.js installation package and does not need to be installed separately.
You can check for the availability of the crypto
module by using the require
function. If the module is available, it will return an object containing the module’s exports. If the module is not available, it will throw an error.
For example:
try {
var crypto = require('crypto');
console.log('crypto module is available');
} catch (err) {
console.log('crypto module is not available');
}
You can also use typeof crypto
to check crypto module availability.
if (typeof crypto !== 'undefined') {
console.log('crypto module is available');
} else {
console.log('crypto module is not available');
}
2. There is also a npm crypto package. This package is not a replacement of the built-in crypto library, it is an addition. It provides additional functionality and flexibility over the built-in crypto library.
If you wish to use the additional functionality provided by the npm package, you can install it using npm in your application and use it along with the built-in crypto library.
Installing Node.Js Crypto Module:
npm install crypto-js --save
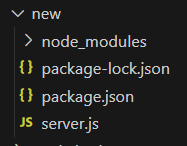
This will install the crypto package to your application and you can use it by requiring it in your code.
const crypto = require('crypto');
It’s important to note that if you’re using the crypto module in a development environment, you should always use the latest version of Node.js, as it may contain security updates and bug fixes.
Uses of the Nodejs Crypto Module
Here are a few examples of the functionality provided by the crypto
module:
1. Hashing
What is Hashing? Hashing is a method to convert a plain text to an encrypted text or cipher text. Hashing is a one-way cryptographic function, meaning that you cannot recover the original text from the cipher text, but you can regenerate the exact cipher text from the original text. This is used for securely storing login password details in the database. It is also used to validate authenticity of files, documents, and data. Some of the widely used hashing algorithms are MD5, RSA, SHA, etc.
You can use the crypto.createHash()
method to create a hash object and then use the .update()
and .digest()
methods to add data to the hash and generate a digest.
const crypto = require('crypto');
const hash = crypto.createHash('sha256');
hash.update('some data to hash');
console.log(hash.digest('hex')); //outputs the hash of 'some data to hash'
2. HMAC
The crypto module also provides a way to create a keyed-hash message authentication code (HMAC) by using the crypto.createHmac()
method.
const crypto = require('crypto');
const hmac = crypto.createHmac('sha256', 'a secret key');
hmac.update('some data to hash');
console.log(hmac.digest('hex')); //outputs the hmac of 'some data to hash'
3. Generating random data
You can use the crypto.randomBytes()
method to generate cryptographically secure random data.
const crypto = require('crypto');
const buf = crypto.randomBytes(16);
console.log(buf.toString('hex')); // outputs a random 16 bytes hexadecimal string
4. Encryption and decryption
Encryption algorithms take data input and a key input to generate a random ciphertext output. Encryption operations are reversible. The reverse of encryption is called decryption. The decryption process take the same secret key (private keys) and ciphertext as input to decipher the original data. An example of encryption/decryption is in messaging apps like WhatsApp where messages and first encrypted before transferring them onto the network and then again decrypted at the readers end to keep messages secure from getting leaked and hacked. Triple DES, AES, RSA Security, Blowfish, Twofish, etc. are some example of encryption/decryption algorithms.
The crypto module provides a way to encrypt and decrypt data using symmetric key ciphers like AES.
const crypto = require('crypto');
const algorithm = 'aes-256-cbc';
const password = 'my secret key';
const key = crypto.scryptSync(password, 'salt', 32);
const iv = Buffer.alloc(16, 0);
const cipher = crypto.createCipheriv(algorithm, key, iv);
let encrypted = cipher.update('some data to encrypt', 'utf8', 'hex');
encrypted += cipher.final('hex');
console.log(encrypted); // outputs the encrypted data
const decipher = crypto.createDecipheriv(algorithm, key, iv);
let decrypted = decipher.update(encrypted, 'hex', 'utf8');
decrypted += decipher.final('utf8');
console.log(decrypted); // outputs the decrypted data
These are just a few examples of the functionality provided by the crypto
module in Node.js.
The module provides many other cryptographic functions as well, such as creating digital signatures, Diffie-Hellman key exchange and various crypto primitives like RSA, ECDSA, and EdDSA.
Commonly used functions in the crypto module
crypto.createHash(algorithm)
: creates a hash object that can be used to generate a hash (also known as a digest) of some data using the specified algorithm. The supported algorithms are sha1, sha256, sha512, md5, etc.
crypto.createHmac(algorithm, key)
: creates a Hmac object that can be used to generate a hash-based message authentication code (HMAC) using the specified algorithm and key. The supported algorithms are sha1, sha256, sha512, md5, etc.
crypto.createSign(algorithm)
: creates a signing object that can be used to sign data using the specified algorithm. The supported algorithms are RSA-SHA1, RSA-SHA256, RSA-SHA512, etc.
crypto.createVerify(algorithm)
: creates a verification object that can be used to verify signed data using the specified algorithm. The supported algorithms are RSA-SHA1, RSA-SHA256, RSA-SHA512, etc.
crypto.createCipher(algorithm, password)
: creates a cipher object that can be used to encrypt data using the specified algorithm and password. The supported algorithms are AES-128-CBC, AES-192-CBC, AES-256-CBC, etc.
crypto.createDecipher(algorithm, password)
: creates a decipher object that can be used to decrypt data using the specified algorithm and password. The supported algorithms are AES-128-CBC, AES-192-CBC, AES-256-CBC, etc.
crypto.createDiffieHellman(primeLength)
: creates a Diffie-Hellman key exchange object that can be used to securely exchange keys. The primeLength can be any value between 512 and 8192 in increments of 64.
crypto.createECDH(curve)
: creates a Elliptic Curve Diffie-Hellman (ECDH) key exchange object that can be used to securely exchange keys. The curve can be any one of the named curves such as ‘secp521r1’, ‘secp384r1’, ‘prime256v1’, etc.
crypto.createDecipheriv(algorithm, key, iv)
: creates a decipher object that can be used to decrypt data using the specified algorithm, key and initialization vector (iv).
crypto.createHash(algorithm, options)
: creates a hash object that can be used to generate a hash (also known as a digest) of some data using the specified algorithm and options.
crypto.randomBytes(size)
: generates cryptographically strong pseudo-random data of the specified size. It is commonly used to generate random keys or nonces.
crypto.randomFill(buffer, offset, size)
: fills the specified buffer with cryptographically strong pseudo-random data starting at the specified offset and continuing for the specified size.
crypto.pbkdf2Sync(password, salt, iterations, keylen, digest)
: generates a derived key using the PBKDF2 algorithm, which is a password-based key derivation function.
crypto.scryptSync(password, salt, keylen, options)
: generates a derived key using the scrypt algorithm, which is a password-based key derivation function designed to be more computationally expensive than PBKDF2.
crypto.getCiphers()
: returns an array of the names of the supported ciphers.
crypto.getHashes()
: returns an array of the names of the supported hash algorithms.
crypto.getDiffieHellman(group_name)
: returns a predefined Diffie-Hellman key exchange object.
crypto.createCredentials(options)
: creates a credentials object that can be used to establish secure connections using the tls module.
crypto.createECDH(curve)
: creates a Elliptic Curve Diffie-Hellman (ECDH) key exchange object that can be used to securely exchange keys.
crypto.publicEncrypt(key, buffer)
: encrypts the given buffer with the given public key.
crypto.privateDecrypt(key, buffer)
: decrypts the given buffer with the given private key.
crypto.generateKeyPairSync(type, options)
: generates a public/private key pair in synchronous way.
crypto.createCipheriv(algorithm, key, iv)
: creates a cipher object that can be used to encrypt data using the specified algorithm, key and initialization vector (iv).
crypto.createCredentials(options)
: creates a credentials object that can be used to establish secure connections using the tls module.
That’s all for this guide. Hopefully you found it useful! 🔮