Table of Contents
Overview
The crypto.constants
object in Node.js provides a collection of constant values that can be used to configure various cryptographic operations. These constants are used as options for methods such as crypto.createCipher()
, crypto.createDecipher()
, crypto.createDiffieHellman()
and others.
Constants provided by the crypto.constants
object
1. crypto.constants.RSA_NO_PADDING
This constant can be used as an option for the crypto.privateEncrypt()
method to specify that the RSA encryption algorithm should not use padding.
const crypto = require('crypto');
const privateKey = `-----BEGIN RSA PRIVATE KEY-----
MIIEogIBAAKCAQEAsf7Z/8GKW/7vz2P9XN/y3C1iLlJ6v5x5j5v5r5f5x5j5v5r5
f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5
x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5
j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5
-----END RSA PRIVATE KEY-----`;
const data = 'This is a secret message';
const encrypted = crypto.privateEncrypt(
{key: privateKey, padding: crypto.constants.RSA_NO_PADDING},
Buffer.from(data)
);
console.log(encrypted.toString('hex'));
🔮 Explore: Complete Guide to Node.js Crypto Module
2. crypto.constants.RSA_PKCS1_PADDING
This constant can be used as an option for the crypto.privateEncrypt()
method to specify that the RSA encryption algorithm should use PKCS1 padding.
const crypto = require('crypto');
const privateKey = `-----BEGIN RSA PRIVATE KEY-----
MIIEogIBAAKCAQEAsf7Z/8GKW/7vz2P9XN/y3C1iLlJ6v5x5j5v5r5f5x5j5v5r5
f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5
x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5
j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5
-----END RSA PRIVATE KEY-----`;
const data = 'This is a secret message';
const encrypted = crypto.privateEncrypt(
{key: privateKey, padding: crypto.constants.RSA_PKCS1_PADDING},
Buffer.from(data)
);
console.log(encrypted.toString('hex'));
In this example, we used the RSA_PKCS1_PADDING constant to specify that the RSA encryption algorithm should use PKCS1 padding.
👉 Popular course: Create Your First ERC-20 Token in Cairo on Starknet
3. crypto.constants.RSA_PKCS1_OAEP_PADDING
This constant can be used as an option for the crypto.privateEncrypt()
method to specify that the RSA encryption algorithm should use RSA_PKCS1_OAEP padding.
const crypto = require('crypto');
const privateKey = `-----BEGIN RSA PRIVATE KEY-----
MIIEogIBAAKCAQEAsf7Z/8GKW/7vz2P9XN/y3C1iLlJ6v5x5j5v5r5f5x5j5v5r5
f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5
x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5
j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5v5r5f5x5j5
-----END RSA PRIVATE KEY-----`;
const data = 'This is a secret message';
const encrypted = crypto.privateEncrypt(
{key: privateKey, padding: crypto.constants.RSA_PKCS1_OAEP_PADDING},
Buffer.from(data)
);
console.log(encrypted.toString('hex'));
In this example, we used the RSA_PKCS1_OAEP_PADDING constant to specify that the RSA encryption algorithm should use RSA_PKCS1_OAEP padding.
As you can see, the constants provided by the crypto.constants
object can be used to configure various cryptographic operations, such as specifying the padding mode to be used in RSA encryption.
These constants are not limited to RSA encryption, but also can be used in other cryptographic operations like Diffie-Hellman key exchange, ECDH and others.
It’s important to note that, these are just examples of how the crypto.constants can be used in real-life applications, but the usage is not limited to these specific examples, the constants can be used in various other scenarios as well.
📌 Bookmark this: What is the createDecipheriv() method in Node.js crypto?
Uses and advantages of crypto.constants
crypto.constants
in Node.js provide a collection of constant values that can be used to configure various cryptographic operations. These constants provide a way to specify options and settings for cryptographic operations in a consistent and readable manner.
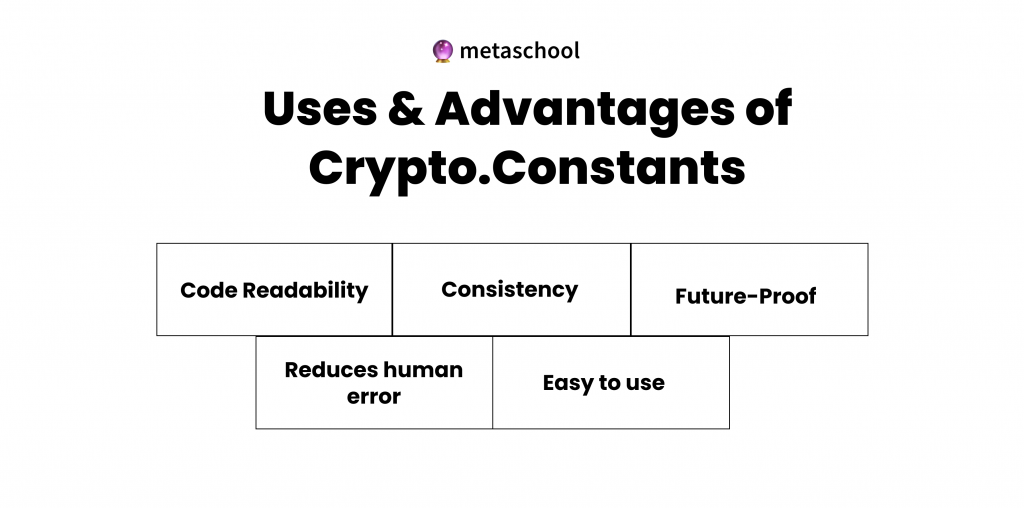
1. Code readability
Using constants instead of hard-coded values makes the code more readable and self-explanatory. For example, it is easier to understand the purpose of a line of code that uses the crypto.constants.RSA_PKCS1_OAEP_PADDING
constant than a line of code that uses a hard-coded value such as 4
to specify the padding mode.
2. Consistency
By using constants, it is easier to ensure consistency across different parts of the codebase. This can help to prevent errors and improve the overall maintainability of the code.
3. Future-proof
Cryptographic algorithms and standards are constantly evolving, and using constants makes it easier to adapt to these changes. For example, if a new padding mode is introduced, the constant can be updated in a single location, rather than having to update every instance of the hard-coded value throughout the codebase.
☀️Explore this: Build a Code Translator Using NextJS and OpenAI API
4. Reducing human error
Using constants reduces the possibility of human error, when used instead of hard-coded values, this means less possibility of misconfiguring the cryptographic operations which can lead to security vulnerabilities.
5. Easy to use
crypto.constants
are easy to use, you don’t need to remember numeric values or string values, you just use the constants, this makes it easy to understand and implement cryptographic operations in your application.
Hopefully this helped you understand the topic! Share it with a friend and help them out too. 🤩
FAQs
How does Crypto.constants in Node.js enhance cryptographic security?
It is a module in Node.js that enhances the overall cryptographic security by providing a set of predefined constants that are also related to cryptographic operations. The main role played by the constants is to ensure that it helps in enforcing strong encryption, secure key exchange, secure hashing, and that the applications adhere to the recommended security measures. This, in turn, enhances the security.
What are the advantages of Crypto.constants over manual constant definition?
Some advantages Crypto.constants has over manual constant definition are accuracy, consistency, and best practices to ensure security.