Table of Contents
Overview
Concatenating the strings means combining two strings to make one string. In Solidity, concatenating a string is a complex step. Like in some programming languages, we have a straightforward method: simply use the “+” sign and concatenate a string. But in Solidity, we can’t do this.
In Solidity, we have different built-in functions that offer string concatenation. In this article, we will discuss two functions one by one. So, let’s dive in.
🔥 Check this course out: Create Your Own Ethereum Token in Just 30 Mins
#1: Using the abi.encodePacked()
function
In Solidity, some built-in functions can be called without importing any other contract. These functions are ABI-encoded functions. To concatenate the strings we can use abi.encodePacked()
function. This function simply takes two strings and returns a single-byte type array which we convert to string to create one concatenated string.
Let’s look at an example.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
contract ExampleContract {
function concatenate(string memory str1, string memory str2) public pure returns (string memory)
{
// Concatenating the strings
return string(abi.encodePacked(str1, str2));
}
}
Explanation
Let’s look at what we did in the example code.
- We created a contract named
ExampleContract
. - We created a pure public function named
concatenate
that takes two memory-type string arguments and returns a memory-type string. - We return a string inside the function by concatenating the strings using the
abi.encodePacked()
function and then type-casting it to the string using thestring()
function.
🔥 Check this course out: Write Your First Solidity Smart Contract on Ethereum
Output
Let’s look at how the output will look after running the code in the RemixIDE.
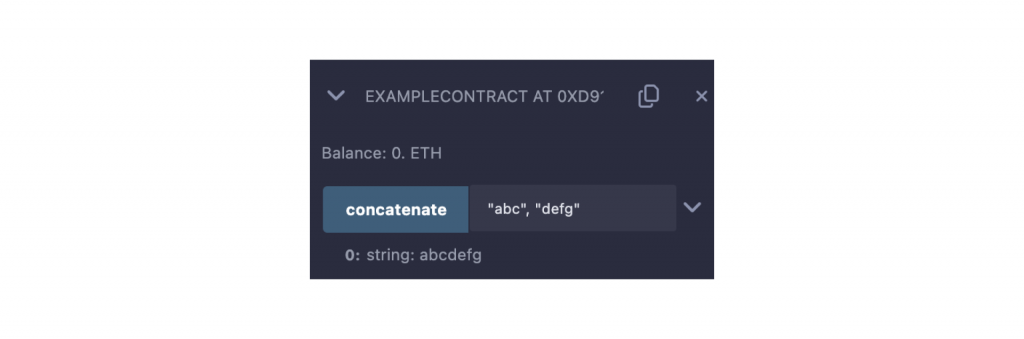
#2: Using the string.concat()
function
Same as abi.encodePacked()
function, Solidity’s newer versions offer a built-in function called string.concat()
that you can use to perform string concatenation without importing any other contract. The string.concat()
function takes two string arguments and returns one concatenated string.
Let’s look at an example.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
contract ExampleContract {
function concatenate(string memory str1, string memory str2) public pure returns (string memory)
{
// Concatenating the strings
return string.concat(str1, str2);
}
}
Explanation
Let’s look at what we did in the example code.
- We created a contract named
ExampleContract
. - We created a pure public function named
concatenate
that takes two memory-type string arguments and returns a memory-type string. - Inside the function, we return a string by concatenating the two strings using the
string.concat()
function.
Output
Let’s look at how the output will look after running the code in the RemixIDE.

Conclusion
Concatenating the strings has become a straightforward process now as Solidity has evolved using the newer versions. There is also an iterative approach to concatenate the strings, but it is long and a bit tricky to implement. So, it is better to use the built-in functions and make your life easy peasy lemon squeezy.
Try it out and let us know how it went by tagging Metaschool on Social Media.
Follow us on –
🔮Twitter – https://twitter.com/0xmetaschool
🔗LinkedIn – https://www.linkedin.com/company/0xmetaschool/